Traffic Light¶
Description:¶
The traffic light is a project that runs on Raspberry Pi. It consists of two cross-road lights; each consists of three light colors: red, yellow, and green. Its function is similar to any traffic light in real life. It facilitates and organizes car movement and reduces the risk of accidents.
Learning Objectives:¶
The traffic light project helps students learn and apply the following:
- Coding, electronics, robotics science, and physical science through a hands-on application that can be directly related to real life situations
- Synchronization and timing
- The implementation of the engineering design process
- Application of theoretical concepts to real life situations
Materials Needed:¶
- Raspberry Pi with micro SD card and Raspbian
- Jumper wires
- 6 LED’s; 2 of each of the colors red, yellow, green
- Resistors 300Ω or similar
- Breadboard
- Glue gun and glue sticks
- Cutter blades or scissors
- Thin sturdy cardboard (poster or portfolio cardboard)
Setup and Functionality:¶
The Traffic light works in an alternating pattern. When the red light is on for one of them, the other one is green. This will allow movement of cars on one of the roads at one time on the cross road in order to avoid accidents and facilitate the movement of cars. When it is the turn of the next path, the yellow light will turn on for few seconds alerting cars to stop on the red light which will turn on right after the yellow. The green light will directly turn on for the second road. The cycle goes on and on. The timing of the lights (red and green) on each road is determined by the amount of traffic on each road.
Circuitry and Electronics:¶
The digrams below show all the wiring and connections of all the componenets used in the traffic light:
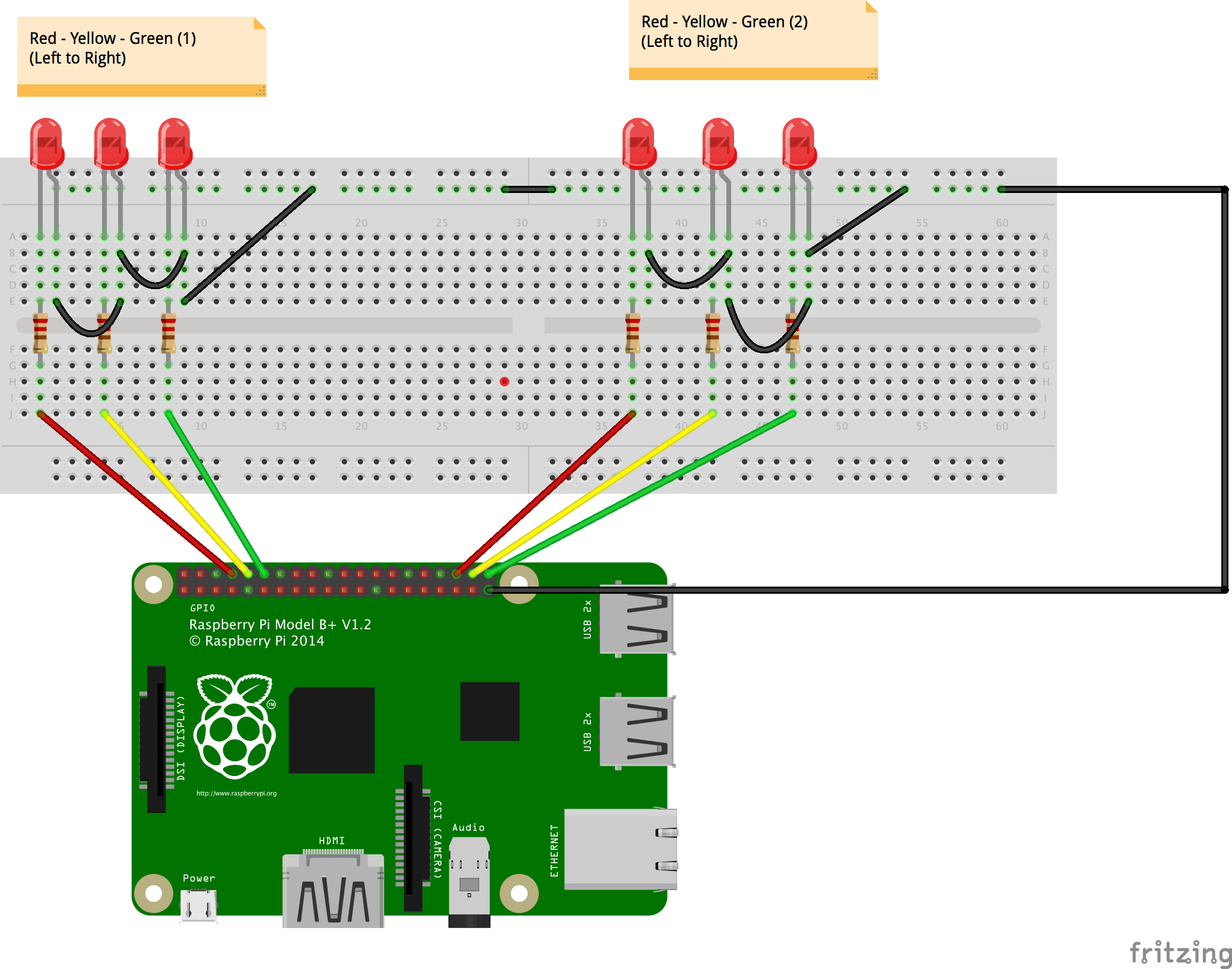
Figure 1: shows the wiring and connections of all the components of the Traffic Light project
Warning
The LED has positive and negative terminals. The longer pin should be connected the GPIO of the Raspberry Pi which is considered as the positive pole, and the shorter pin should be connected to the ground pin (Gnd) on the Raspberry Pi. The LED will not light in case the wires are switched.
Programming:¶
The Traffic Light has been programmed using Python language:
import RPi.GPIO as GPIO
import time
def TurnOffAllLights():
stateOfFirstTrafficLight(green=False, yellow=False, red=False)
stateOfSecondTrafficLight(green=False, yellow=False, red=False)
def stateOfFirstTrafficLight(green, yellow, red):
GPIO.output(firstGreen, green)
GPIO.output(firstYellow, yellow)
GPIO.output(firstRed, red)
def stateOfSecondTrafficLight(green, yellow, red):
GPIO.output(secondGreen, green)
GPIO.output(secondYellow, yellow)
GPIO.output(secondRed, red)
GPIO.setmode(GPIO.BOARD)
TIME_BEFORE_SWITCH = 1
# TrafficLights 1
firstRed = 8
firstYellow = 10
firstGreen = 12
# TrafficLights 2
secondRed = 36
secondYellow = 38
secondGreen = 40
GPIO.setup(firstRed, GPIO.OUT)
GPIO.setup(firstYellow, GPIO.OUT)
GPIO.setup(firstGreen, GPIO.OUT)
GPIO.setup(secondRed, GPIO.OUT)
GPIO.setup(secondYellow, GPIO.OUT)
GPIO.setup(secondGreen, GPIO.OUT)
# make sure all lights are turned off at the beginning
TurnOffAllLights()
try:
while True:
stateOfFirstTrafficLight(green=True, yellow=False, red=False)
stateOfSecondTrafficLight(green=False, yellow=False, red=True)
time.sleep(TIME_BEFORE_SWITCH)
stateOfFirstTrafficLight(green=False, yellow=True, red=False)
stateOfSecondTrafficLight(green=False, yellow=False, red=True)
time.sleep(TIME_BEFORE_SWITCH)
stateOfFirstTrafficLight(green=False, yellow=False, red=True)
stateOfSecondTrafficLight(green=True, yellow=False, red=False)
time.sleep(TIME_BEFORE_SWITCH)
stateOfFirstTrafficLight(green=False, yellow=False, red=True)
stateOfSecondTrafficLight(green=False, yellow=True, red=False)
time.sleep(TIME_BEFORE_SWITCH)
# If CTRL+C is pressed the main loop is broken
except KeyboardInterrupt:
TurnOffAllLights()
Science Concepts and Skills:¶
The Traffic Light project helps students learn and apply the Engineering Design Process, learn how to connect electric and electronic circuits, work in teams, solve problems, and relate their learned concepts to real life situations. The timing of the lights and the choice of the location should be based on site observations. The number of cars passing at each path should be observed and data should be collected to determine proper timing for each road light. Some streets are more crowded than others. This should be taken into consideration when planning for the project.
In addition, this project is a prototype sample that uses only two sets of lights. More light sets can be added depending on the number of roads on the crossroad.