Vibrating Creature¶
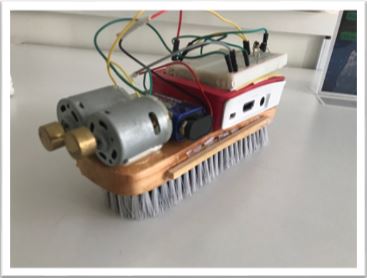
Description¶
The vibrating creature is a project that runs on Raspberry Pi. It’s simply a shoe brush used for brushing shoes that moves in a linear motion due to a vibrating system built inside of it. The vibrating creature is designed and constructed based on the engineering design process that is followed in the Coder-Maker program.
Learning Objectives¶
The vibrating creature helps students learn and apply the following:
- Force and linear motion
- Friction
- The implementation of engineering design process
- Application of theoretical concepts to real life situations
- Coding, electronics, and physical science through a hands-on application that
Materials Needed¶
- Raspberry Pi with microSD card and Raspbian
- Two 9V DC motors
- Jumper wires
- Two transistors of type 2N4401
- Two diodes of type 1N5404
- A breadboard
- Cutter blades
- Glue gun and glue sticks
- Power Bank
- Shoe brush (check the image below for an example brush)
- Two Resistors (300Ω or similar)
- Thick white cardboard
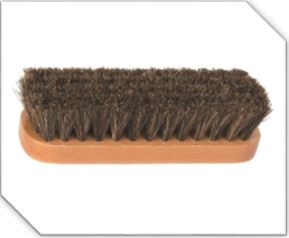
Setup and Functionality¶
The vibrating creature is a project that is controlled by two DC motors. When the motor is spinning, the round piece’s weight is applying a pushing force to the brush causing the whole object to vibrate thus allowing it to move forward in a linear path.
Circuitry and Electronics:¶
The diagrams below show all the wiring and connections of all the components used in the Vibrating Creature:
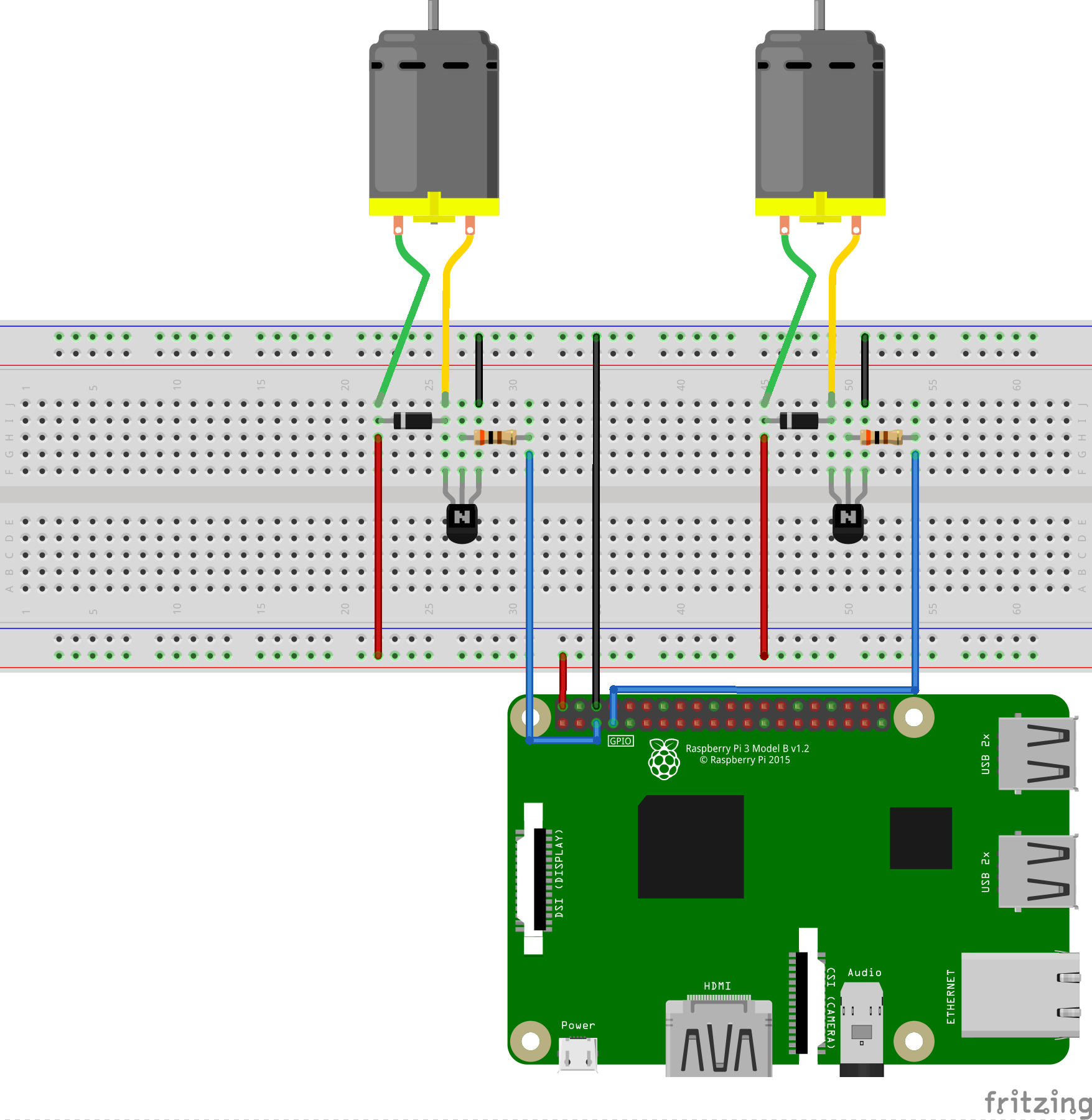
Programming¶
import RPi.GPIO as GPIO
from time import sleep
GPIO.setmode(GPIO.BOARD)
GPIO.setwarnings(False)
fan=7
dc=5
GPIO.setup(dc,GPIO.OUT)
GPIO.setup(fan, GPIO.OUT)
pd= GPIO.PWM(dc,100)
pf=GPIO.PWM(fan,100)
try:
while True:
c=input("Move the object? If yes, type 0:\n" ) #The motor is started when the number 0 is given as input
if c==0:
print('Object moving')
pd.start(100)
pf.start(100)
sleep(5)
pf.stop()
pd.stop()
else: #Condition is set as for any other input the creature won’t move
print('Object stopped')
sleep(1)
except KeyboardInterrupt:
pf.stop()
pd.stop()
Science Concepts and Skills¶
The movement of the object is the result of two things, the vibration and the directed push of the fan. It is important to know that one could not have worked without the other. The fan alone cannot push the creature to move. Why? Since we are dealing with horizontal motion on a surface, there are two main forces affecting motion. The force that pushes the cube forward created by the fan, and the force of friction which acts against the motion (backwards). When the cube is at rest and not vibrating, the force of friction is greater than the forward force which prevents the object from moving forward. When the object vibrates, vibrations cause it to lift up from the surface and then go back to its original position on the surface.

When in a lifted position, the friction force between the surface and the cube is negligible which results in a greater forward force, which causes the cube to move forward. When the cube gets back on the surface, the force of friction increases resulting in no motion. That is why it is observed to be moving in a jittering motion. The nature of the surface and the weight of the object affect the force of friction. The rougher the surface and the heavier the moving object, the bigger the force of friction and vice versa. Check out this Visual Resource.
Flex your brain! How can this concept be applied to reduce the fuel consumption of engine driven vehicles over several types of surfaces?