The Automated Garage (Student Version)¶
Description¶
The Garage is a project that runs on Raspberry Pi. It has a gate that opens and closes to allow entry and exit of cars.The gate opens automatically when sensing a car. The Garage is designed and constructed based on the engineering design process that is followed in the Coder-Maker program.
Materials Needed:¶
- Raspberry Pi with micro SD card and Raspbian
- Two ultrasonic sensors
- Micro servo motor
- Jumper wires
- Resistors 300Ω or similar
- Breadboard
- Glue gun and glue sticks
- Cutter blades or scissors
- Thin sturdy cardboard (poster or portfolio cardboard)
Setup and Functionality:¶
The garage is a system that is controlled by two ultrasonic sensors that are connected to a micro servo motor. The gate consists of a bar that rises up to an angle of 45° from horizontal position to open and goes back to its original position to close. The opening and closure of the bar is operated by the micro servo motor. The gate movement is fully automated. Two ultrasonic sensors are installed, one al the entrance at the level of the bar and one on the exit wall of the garage. The function of the one facing the bar is to open the bar automatically as well as preventing it from closing if a car is exiting or entering the garage and is still stopping under the bar in order to prevent damage. The function of the one on the exit wall is to give an order to the micro servo motor to open the bar automatically allowing the car to exit.
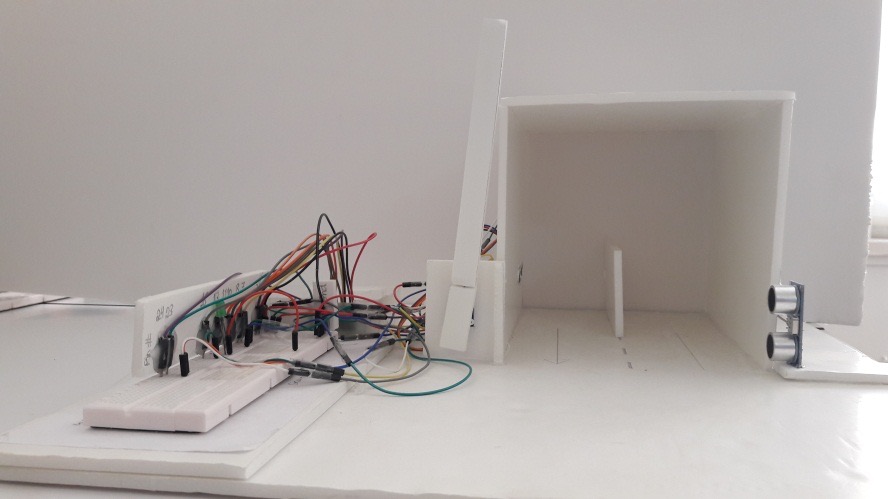
Figure 1: Shows a picture of the constructed Garage
Circuitry and Electronics:¶
The diagrams below show all the wiring and connections of all the components used in the Garage:
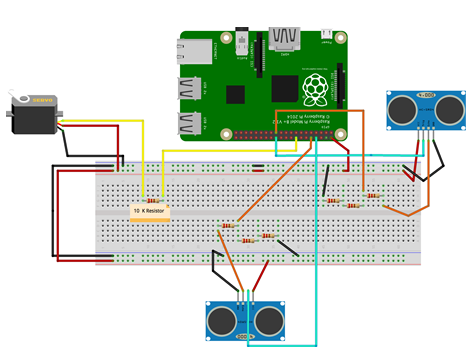
Figure 2: shows the wiring and connections of all the components of the Garage project
Warning
The micro servo motor does not consume much current and can be operated directly on the Raspberry Pi 6V power output. Make sure to use the same type of motor as other types might require a current that the Raspberry Pi cannot handle unless a transistor is added to the circuitry or an external power supply.
Programming¶
The Garage is programmed using Python language:
Code of the automatic garage
import RPi.GPIO as GPIO
import time
trigOpen = 24
echoOpen = 23
trigClose = 8
echoClose = 10
servoM = 16
GPIO.setwarnings(False)
GPIO.setmode(GPIO.BOARD)
GPIO.setup(trigOpen, GPIO.OUT)
GPIO.setup(echoOpen, GPIO.IN)
GPIO.output(trigOpen, False)
GPIO.setup(trigClose, GPIO.OUT)
GPIO.setup(echoClose, GPIO.IN)
GPIO.output(trigClose, False)
GPIO.setup(servoM, GPIO.OUT)
servo = GPIO.PWM(servoM, 50)
distance = 7
def reading(trig, ech):
GPIO.output(trig, False)
time.sleep(0.3)
GPIO.output(trig, True)
time.sleep(0.001)
GPIO.output(trig, False)
signalOff = time.time()
while GPIO.input(ech) == 0:
signalOff = time.time()
while GPIO.input(ech) == 1:
signalOn = time.time()
timePassed = signalOn - signalOff
distance = timePassed * 17150
distance = round(distance, 2)
return distance
try:
while True:
time.sleep(0.5)
distance = reading(trigOpen, echoOpen)
if(distance < 5):
isOpen = 1
while(isOpen == 1):
print("should open")
servo = GPIO.PWM(servoM, 50)
servo.start(50)
servo.ChangeDutyCycle(12.5)
time.sleep(5)
distanceClose = reading(trigClose, echoClose)
if distanceClose < 10:
print("can not close the gate")
else:
servo.ChangeDutyCycle(7.2)
isOpen = 0
time.sleep(1)
servo.stop()
except KeyboardInterrupt:
servo.stop()
GPIO.cleanup()