The Walking Creature (Student Version)¶
Description¶
The walking creature is a project that is programmed on the Raspberry Pi to walk on six legs. It lifts one side of its legs at one time in order to maintain forward movement. The walking creature is designed and constructed based on the engineering design process that is followed in the Coder-Maker program.
Materials Needed¶
- Raspberry Pi with micro SD card and Raspbian
- Two micro servo motors
- Jumper wires
- Breadboard
- Bendable metal wires
- Glue gun and glue sticks
- Cutter blades or scissors
- Thin sturdy cardboard (poster or portfolio cardboard)
Setup and Functionality¶
The walking creature is a 30*20cm project that is made up of cardboard, 2 micro servo motors, and metal wires . It is able to move forward by the action of the 2 motors. One motor is attached to the front and back legs, which are synchronized, and the middle legs are operated by the action of the second micro servo motor. The front and back legs move forward and backward. This ensures forward movement only due to the action of the middle legs is to raise the side where the legs are moving forward to present the whole creature from moving in place. This will allow only the legs that are pushing forward to touch the floor.
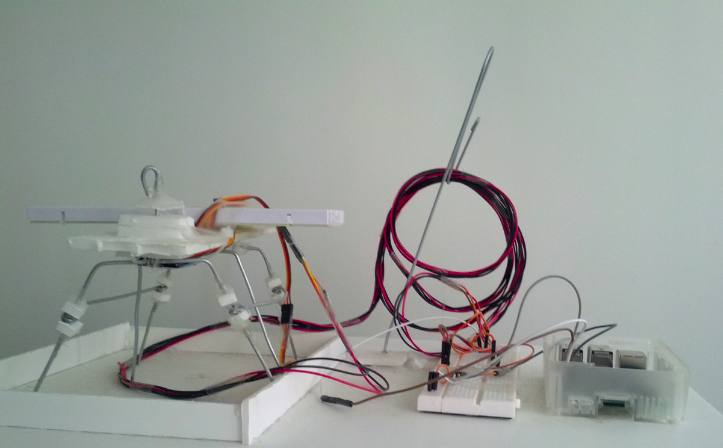
Figure 1: shows a picture of the constructed project
Circuitry and Electronics:¶
The diagrams below show all the wiring and connections of all the components used in the walking creature:
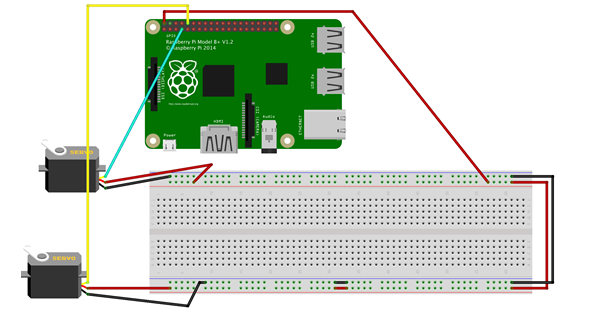
Warning
The micro servo motor does not consume much current and can be operated directly on the Raspberry Pi 6V power output. Make sure to use the same type of motor as other types might require a current that the Raspberry Pi cannot handle unless a transistor is added to the circuitry or an external power supply.
Programming¶
The walking creature is programmed using Python language:
Code of the walking creature
import time
import RPi.GPIO as GPIO
GPIO.setmode(GPIO.BOARD)
firstServo = 7
secondServo = 10
GPIO.setup(firstServo, GPIO.OUT)
GPIO.setup(secondServo, GPIO.OUT)
pwm = GPIO.PWM(firstServo, 50)
gwm = GPIO.PWM(secondServo, 50)
pwm.start(7.5)
gwm.start(7.5)
try:
while True:
pwm.ChangeDutyCycle(2)
gwm.ChangeDutyCycle(2)
time.sleep(0.5)
pwm.ChangeDutyCycle(5)
gwm.ChangeDutyCycle(3.5)
time.sleep(0.5)
except KeyboardInterrupt:
pwm.stop()
gwm.stop()
GPIO.cleanup()