Distance Tracker¶
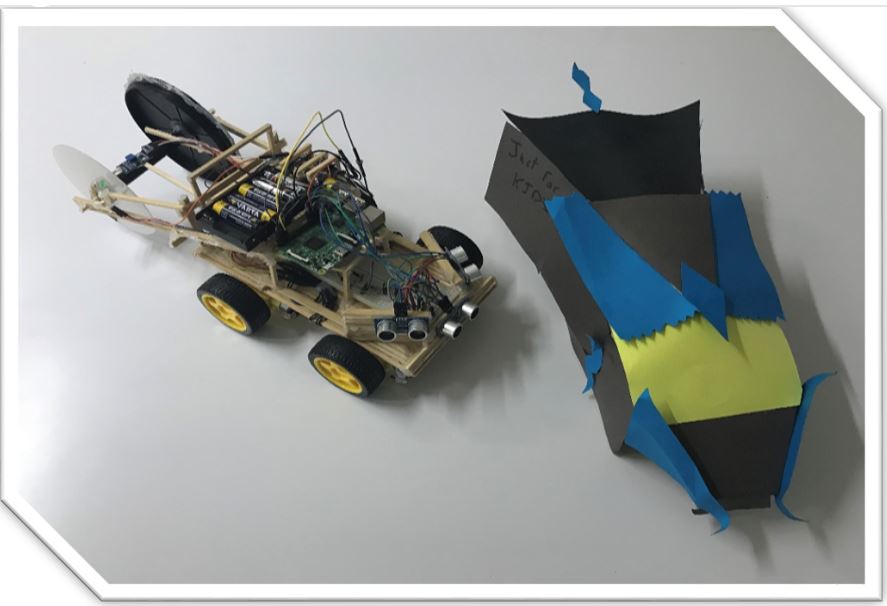
Description¶
This Project can follow a specific path measuring the dimensions of a flat surface.
Learning Objectives¶
The Distance Tracker is a project that helps students learn and apply the following:
- Circular to linear motion
- Force and motion
- Friction
- The implementation of the engineering design process
- Application of theoretical concepts to real life situations
- Coding, electronics, and physical science through a hands-on application that can be directly related to real life situations
Materials Needed¶
- Raspberry pi with micro SD card and Raspbian
- Jumper wires
- Breadboard
- L298n (motor driver)
- Glue gun and glue sticks
- Cutter blades or scissors
- 6 x 1.5v external power supply/batteries
- 4 x 6V Dc gear motor with wheels
- 1 x LED
- 1 x LDR sensor
- 3 x Ultrasonic Sensors
- 10 x resistors
- Wooden sticks
- CD disk with cover
Setup and Functionality¶
The distance tracker is designed in a way that as the vehicle moves forward, it rotates two discs attached at the back of the vehicle. One disk is covered with a rubbery material to increase the friction with the ground (Scientific note: the increase of friction decreases the chance of slipping). A transparent disc with a black line is attached to the first one and rotates at the same speed (see picture below). There are ultrasonic sensors that are used to measure the distance between the moving vehicle and any facing obstacles in the front from the three sides in order to turn and change direction to avoid bumping.
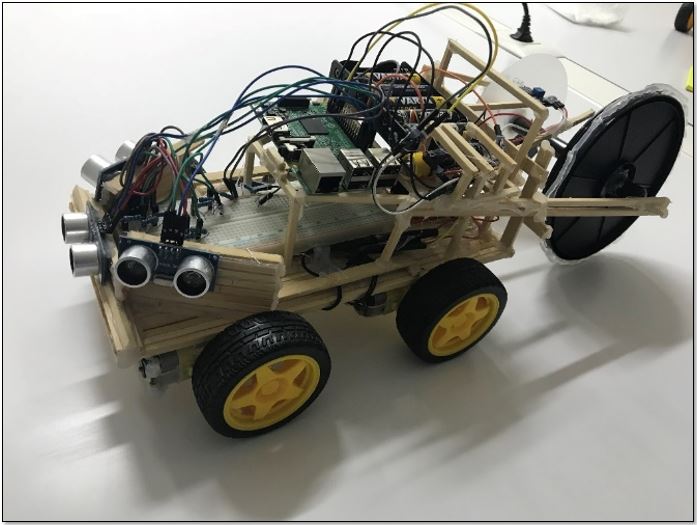
The transparent wheel allows the passage of the green light emitted by the LED. As the light passes through the wheel, it is sensed by a sensor on the other side (LDR sensor). When the black line faces the light, it will block it and will not allow it to pass through. The sensor will consider the light interruption as one turn of the wheel.
Circuitry and Electronics:¶
The diagrams below show all the wiring and connections of all the components used in the Distance Tracker:
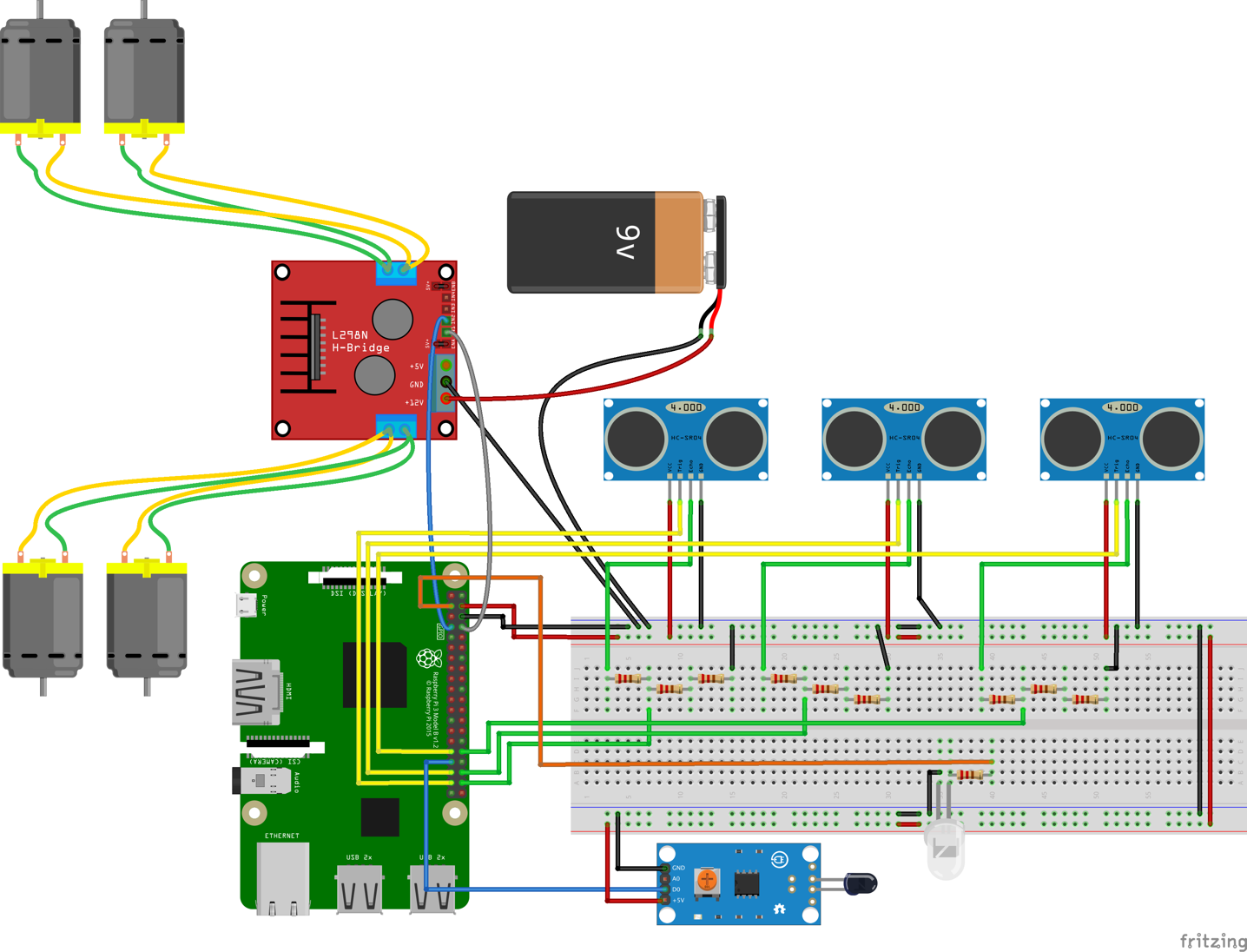
Programming¶
import RPi.GPIO as GPIO
import time
tR=31
eR=32
tL=37
eL=38
tM=35
eM=36
pho=33
counter=0
GPIO.setmode(GPIO.BOARD)
GPIO.setup(tR,GPIO.OUT)
GPIO.setup(eR,GPIO.IN)
GPIO.setup(tL,GPIO.OUT)
GPIO.setup(eL,GPIO.IN)
GPIO.setup(tM,GPIO.OUT)
GPIO.setup(eM,GPIO.IN)
GPIO.setup(7,GPIO.OUT)
GPIO.setup(8,GPIO.OUT)
GPIO.setup(3,GPIO.OUT)
GPIO.output(7,True)
GPIO.output(8,True)
GPIO.output(3,True)
def rc_time (pho):
count=0
GPIO.setup(pho,GPIO.OUT)
GPIO.output(pho,GPIO.LOW)
time.sleep(0.1)
GPIO.setup(pho,GPIO.IN)
while (GPIO.input(pho) == GPIO.LOW):
count +=1
return count
def ultrasonic(trig,ech):
time.sleep(0.2)
GPIO.output(trig, True)
time.sleep(0.0001)
GPIO.output(trig, False)
signalOff = time.time()
while GPIO.input(ech) == 0:
signalOff = time.time()
while GPIO.input(ech) == 1:
signalOn = time.time()
timePassed = signalOn - signalOff
distance = timePassed * 17000
distance = round(distance, 2)
return distance
try:
while True:
distanceR = ultrasonic(tR,eR)
distanceL = ultrasonic(tL,eL)
distanceM = ultrasonic(tM,eM)
print 'Distance R:', distanceR, 'cm'
print 'Distance L:', distanceL, 'cm'
print 'Distance M:', distanceM, 'cm'
while distanceR < 20:
print 'Right Off'
GPIO.output(7,False)
GPIO.output(8,True)
distanceR = ultrasonic(tR,eR)
while distanceL < 25:
print 'Left Off'
GPIO.output(8,False)
GPIO.output(7,True)
distanceL = ultrasonic(tL,eL)
if distanceM < 25:
print 'Center Off'
GPIO.output(7,False)
GPIO.output(8,False)
break
GPIO.output(7,True)
GPIO.output(8,True)
print 'LDR',rc_time(pho)
if rc_time(pho) > 0:
counter = counter + 1
print ' Steps', counter
print ' LENGTH', counter*44 , 'cm'
except KeyboardInterrupt:
GPIO.cleanup()
Science Concepts and Skills¶
A vehicle is designed to measure distance on a linear path using the rotation of a circular disk. The circular rotation of the disk in converted to linear motion based on the diameter of the rotating disk.
To measure the linear distance travelled by each rotation, the following formula can be used: Distance travelled = 2 * π * radius of the disk
The unit of measurement for the distance travelled is the same as that of the radius of the disk. It can be measured in centimeters, meters, or other related units.
As is demonstrated in the video above, as the gear wheel rotates, it allows a metal bar to move in a linear war. The distance travelled by the metal bar depends mainly on the radius of the wheel.
In addition, several materials can be tested and wrapped around the wheel to compare in terms of friction. Comparison can take into consideration the most accurate reading for measuring linear distance based on circular motion.
Flex your brain! How can we use this same device to measure its linear velocity?