The Buoyant Device¶
Description¶
The buoyant device is a system that controls the movement of an object upward and downward in a liquid. It consists of a syringe that opens and closes to pump air in and out of the immersed object by the action of two gear motors. The arrows on the keyboard manually control the amount of air pumped in or out of the object. When the syringe reaches its limit, it will stop moving as it is controlled by an ultrasonic sensor.
Learning Objectives¶
The buoyant device is a project that helps students learn and apply the following:
- Weight as a kind of force
- Gravity, mass, volume, and density
- Buoyant force
- Archimedes principle
- The implementation of the engineering design process
- Application of theoretical concepts to real life situations
- Coding, electronics, and physical science through a hands-on application that can be directly related to real life situations
Materials Needed¶
- Raspberry Pi with microSD card and Raspbian
- One ultrasonic sensor
- Two 6V DC gear motors
- Two L293D chips
- Jumper wires
- Hard metal wire or any alternative to hold the motors
- Three Resistors 220Ω or similar
- Breadboard
- Glue gun and glue sticks
- Cutter blades or scissors
- Floating material (styrophoam, plastic, etc)
- A flexible tube, diameter approx 0.5 cm
- A bottle of water
- A big syringe
- Two external power supplies of 6V
- Water container
- Strong non elastic thread
Setup and Functionality¶
The buoyant device is a project that is controlled by two DC motors and an ultrasonic sensor. These motors are attached symmetrically to a syringe using a strong thread. They spin in one direction to push/pull the threads in order to pump air in or out of the bottle Note that the syringe and the bottle are connected with a tube. What this does is remove or add water to the bottle, thus changing its weight. The heavier the bottle is, the deeper it sinks and the lighter it is, the more it floats. As for the ultrasonic sensor, it detects the position of the syringe giving command to the motors to stop pushing or pulling based on its position so it does not fall out of place or push too much breaking the thread.
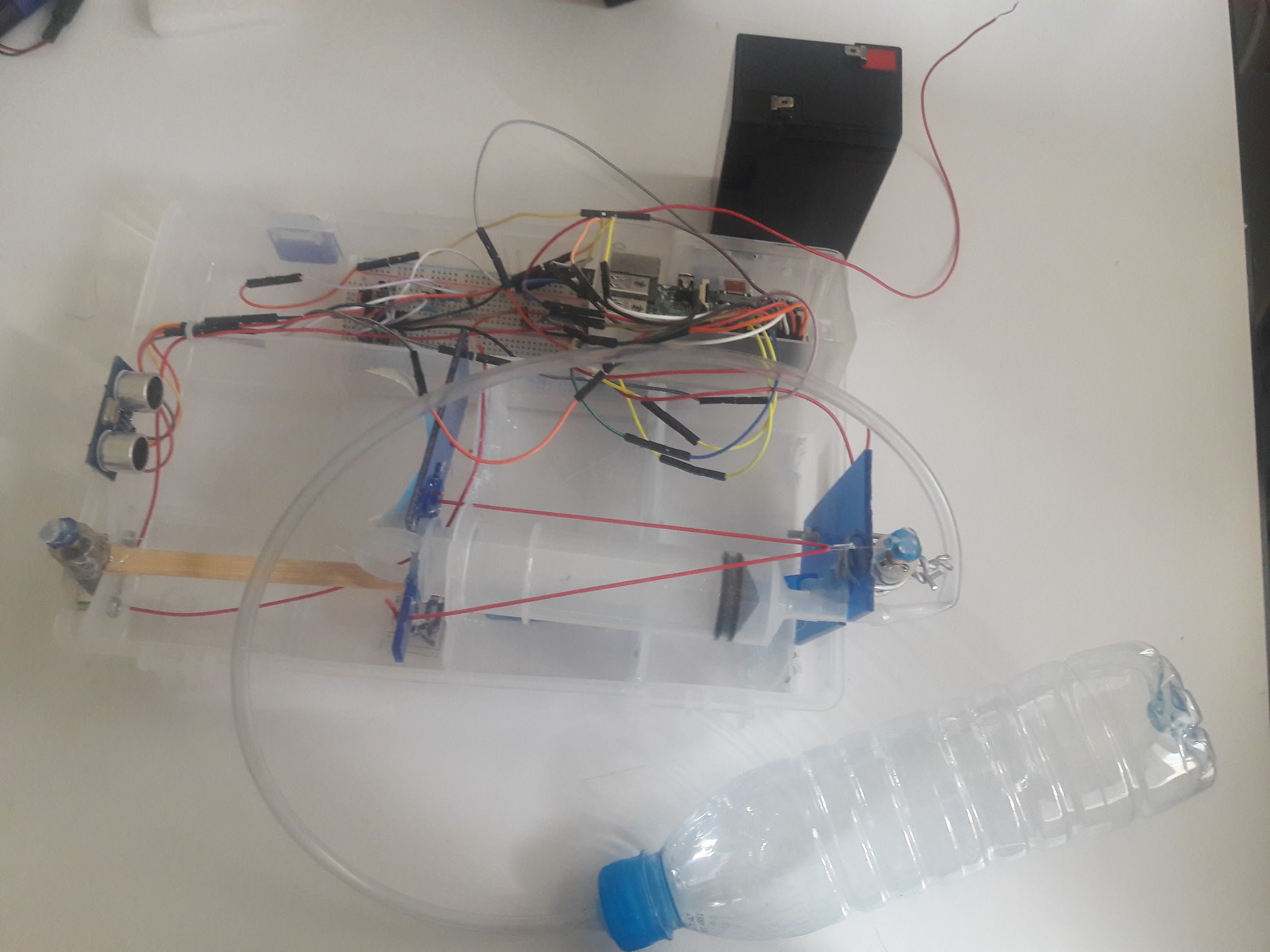
The image above shows the basic setup and the mechanism that pumps water in and out of the bottle. It shows the 2 gear motors, the syringe, the ultrasonic sensor, and the submerged object.
Circuitry and Electronics:¶
The diagram below shows the connections and the circuitry of the 2 gear motors that push and pull the syringe:
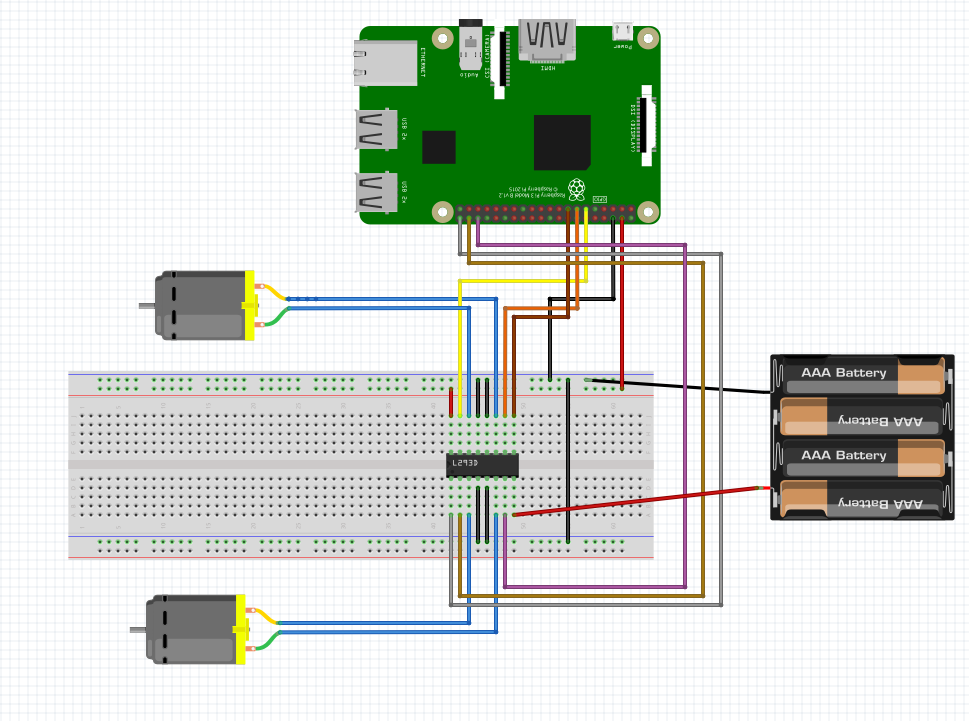
The second diagram below shows the connections and the circuitry of the ultrasonic sensor that controls the movement of the syringe at its limit points:
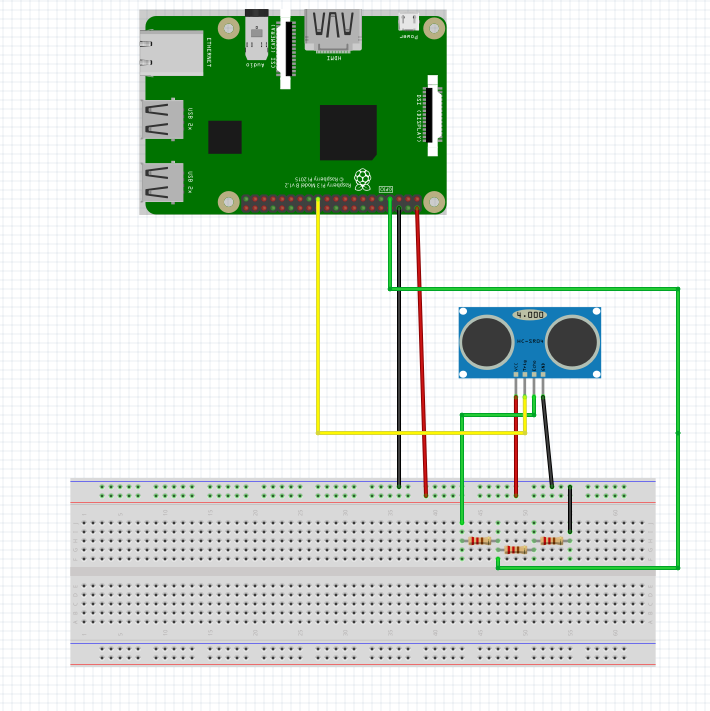
Programming¶
from pykeyboard import PyKeyboardEvent
import RPi.GPIO as GPIO
import time
GPIO.setwarnings(False)
GPIO.setmode(GPIO.BOARD)
#motor1
dcE=40
dcA=38
dcB=36
GPIO.setup(dcE,GPIO.OUT)
GPIO.setup(dcA,GPIO.OUT)
GPIO.setup(dcB,GPIO.OUT)
#motor2
dcE2=15
dcA2=11
dcB2=13
GPIO.setup(dcE2,GPIO.OUT)
GPIO.setup(dcA2,GPIO.OUT)
GPIO.setup(dcB2,GPIO.OUT)
#Echo
GPIO.setup(7,GPIO.IN)
#Trig
GPIO.setup(23,GPIO.OUT)
def reading():
GPIO.output(23,False)
time.sleep(0.3)
GPIO.output(23,True)
time.sleep(0.00001)
GPIO.output(23,False)
while GPIO.input(7)==0:
start=time.time()
while GPIO.input(7)==1:
stop=time.time()
timePassed=stop-start
distance=timePassed*17000
distance=round(distance,2)
print 'Distance: ',distance,' cm'
return distance
class ListenInterrupt(Exception):
pass
class ClickKeyEventListener(PyKeyboardEvent):
def __init__(self):
PyKeyboardEvent.__init__(self)
return
def tap(self, keycode, character, press):
#press in boolean; True for press, False for release
if press:
distance=reading()
if str(keycode) == '111':
#if distance of sensor measurements <max :
if distance > 5.0:
print 'UP'
#loosen the syringe (empty the bottle from water)
GPIO.output(dcE,True)
GPIO.output(dcE2,True)
#Loosen motor1
p=GPIO.PWM(dcB,500)
p.start(70)
#Pull motor2
GPIO.output(dcA2,True)
GPIO.output(dcB2,False)
time.sleep(1)
#Stop motor1
GPIO.output(dcE,False)
GPIO.output(dcA,False)
GPIO.output(dcB,False)
#Stop motor2
GPIO.output(dcE2,False)
GPIO.output(dcA2,False)
GPIO.output(dcB2,False)
elif str(keycode) == '116':
#if distance of sensor measurements >min :
if distance < 10.0 :
print 'Down'
#Pull the syringe (fill the bottle with water)
#Start motor1
GPIO.output(dcE,True)
#Start motor2
GPIO.output(dcE2,True)
##Pull motor1
GPIO.output(dcA,True)
GPIO.output(dcB,False)
##Loosen motor2
p2=GPIO.PWM(dcB2,500)
p2.start(50)
time.sleep(1)
GPIO.output(dcE,False)
GPIO.output(dcE2,False)
GPIO.output(dcB2,False)
GPIO.output(dcB,False)
GPIO.output(dcA,False)
GPIO.output(dcA2,False)
C = ClickKeyEventListener()
try:
C.run()
except ListenInterrupt as e:
print(e.args[0])
Science Concepts and Skills¶
This project relates to Archimedes principle that states the apparent upwardforce (buoyancy) of a body immersed in a fluid is equal to the weight of the displaced fluid. The weight of liquid displaced is the weight of the liquid that has been replaced by the object. The volume of displaced liquid is equal to the volume of the immersed part of the object. When an object is submerged in a liquid, an upward force acts on it with a magnitude equal to the weight of the displaced volume of the liquid. The higher the density of the liquid, the higher the magnitude of the upward force. The upward force is called buoyant force or Archimedes upthrust.
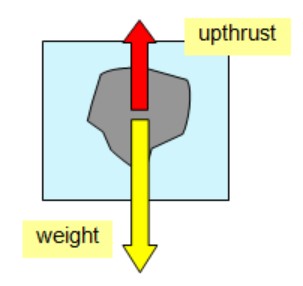
The image above shows the forces acting on an object immersed in a liquid Two forces act on an object in a liquid, its weight and upthrust. The weight of the object is the gravitational pull on its mass. Weight is a force as weight=mass*gravity. Weight is the force that pulls the object downwards. On the other hand, the force that the liquid exerts against the weight is the upthrust. Since there is an upward force on the object, then the weight of the object will be less when immersed in a liquid and is called apparent weight.
Buoyant force is calculated by:
Fb = ρ*g*V
Where:
Fb is the buoyant force in Newton
Ρ is the density of the liquid in kg/m3
g is the gravitational pull in m/s2
V is the volume of displaced liquid in m3
In this project, the bottle has a weight that is added inside it to make it fully immersed in the liquid. Once the air is pumped out of the bottle, its volume becomes less, meaning in return that is will displace less liquid. That results in less weight of the object. I this case, the buoyant force will be greater than the weight of the object resulting in upward movement of the object. If air is pumped into the bottle, the weight will increase resulting in a downward movement as it will be greater than the buoyant force.
Flex your brain! How can you apply buoyancy to build a life saving device?