The Smart Fan¶
Description:¶
The smart fan is a device that runs on Raspberry Pi. It consists of a rotating piece that revolves on a motor axis and changes speed based on the distance from the objects facing it. This will reduce the amount of air current given by the fan as the person comes closer and increases it as the person in front of it goes farther. The fan will stop rotating if the person in front of it comes very close for safety reasons.
Learning Objectives:¶
The Smart Fan is an integrated system that helps students learn and apply the following:
Coding, electronics, robotics science, and physical science through a hands-on application that can be directly related to real life situations.
Motion concepts:
- Sound waves, their properties and speed
- The use of formulas to find distance in relation to speed of sound
- Angular momentum
The implementation of the engineering design process
Application of theoretical concepts to real life situations
Materials Needed:¶
- Raspberry Pi with micro SD card and Raspbian
- One ultrasonic sensor
- One 6V simple DC motors
- Diode
- Transistor
- Jumper wires
- Resistors 300Ω or similar
- Breadboard
- Glue gun and glue sticks
- Cutter blades or scissors
- Thin sturdy cardboard (poster or portfolio cardboard)
Setup and Functionality:¶
The Smart Fan runs on Raspberry Pi and uses a simple DC rotating motor and an ultrasonic sensor. The speed of the rotation of the fan is controlled by the ultrasonic sensor. It is programmed to slow down as the distance between any object and the sensor increases and vice versa. The fan will fully stop rotating if an object comes very close to the sensor.
Circuitry and Electronics:¶
The diagrams below show all the wiring and connections of all the componenets used in the Smart Fan:
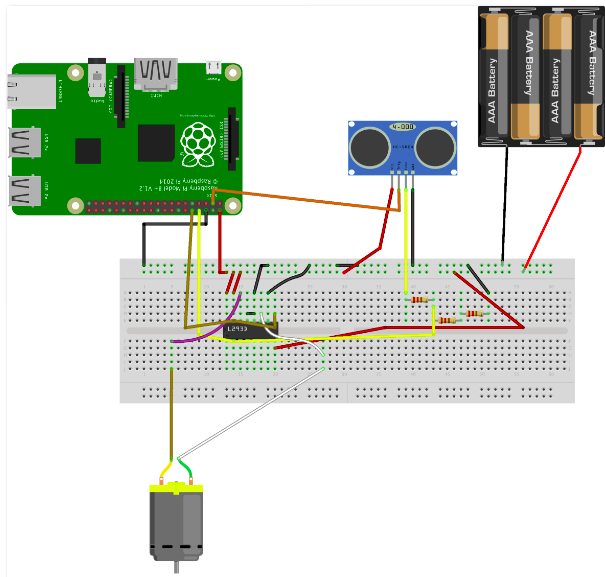
Figure 1: shows the wiring and connections of all the components to the Raspberry Pi.
The function of the transistor is to amplify the current that is produced by Raspberry Pi voltage output GPIO. The 6V simple DC motor requires more current than that provided by the Raspberry Pi. In order to provide the required current, a transistor is connected to the circuit. At the same time, it is essential to connect a diode. Diodes allow current to move only in one direction and prevent it from flowing back. After turning the fan off, the motor keeps rotating for a while before it comes to a stop. During this time, the motor is acting like a generator, thus producing electricity. The diode will prevent the current produced from damaging the GPIO voltage output of the Raspberry Pi.
Warning
Proper attention should be given to the wiring of the diode and transistor. Make sure to follow the diagram above. Improper connection of the transistor will lead to improper functioning of the motor. In addition, reversing the wiring of the diode will cause the current to stop flowing and the whole device will not function.
Programming:¶
The Smart Fan has been programmed using Python language:
Code of the smart fan
import RPi.GPIO as GPIO
import time
def reading(trig, ech):
GPIO.output(trig, False)
time.sleep(0.3)
GPIO.output(trig, True)
time.sleep(0.001)
GPIO.output(trig, False)
signalOff = time.time()
while GPIO.input(ech) == 0:
signalOff = time.time()
while GPIO.input(ech) == 1:
signalOn = time.time()
timePassed = signalOn - signalOff
distance = timePassed * 17000
return distance
trigger = 3
echo = 8
motor = 10
GPIO.setwarnings(False)
GPIO.setmode(GPIO.BOARD)
GPIO.setup(motor, GPIO.OUT)
GPIO.setup(echo, GPIO.IN)
GPIO.setup(trigger, GPIO.OUT)
pwm = GPIO.PWM(motor, 500)
pwm.start(100)
try:
while True:
distance = reading(trigger, echo)
print distance
if distance < 10:
pwm.ChangeDutyCycle(0)
elif distance < 20:
pwm.ChangeDutyCycle(20)
elif distance < 40:
pwm.ChangeDutyCycle(40)
elif distance < 60:
pwm.ChangeDutyCycle(60)
elif distance < 80:
pwm.ChangeDutyCycle(80)
else:
pwm.ChangeDutyCycle(100)
except KeyboardInterrupt:
pwm.stop()
GPIO.cleanup()
Science Concepts and Skills:¶
The Smart Fan helps students learn and apply the Engineering Design Process, learn how to connect electric and electronic circuits, work in teams, solve problems, and relate their learned concepts to real life situations.
Through the Smart Fan project students will learn to choose and use physics formulas in proper context and relate their findings and solutions to daily life where they start to make sense and do not remain abstract theories.
Sound travels in the form of waves that have specific properties as wavelength and frequency. The wavelength is the distance between two waves (see figure 2), and the frequency is the number of waves in every second measured in Hertz (Hz).
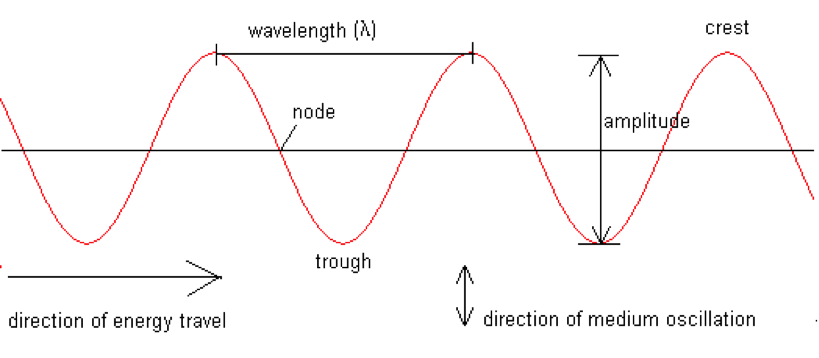
Figure 2: shows a sample of a sound wave with its properties
Speed of a \text{wave}=\text{Frequency}\times \text{Wavelength}
The speed of a sound wave is affected by ambient (air) temperature and it varies based on the formula:
V=331 \frac{m}{s} + 0.6 \frac{m}{s °C} \times \text{Temperature in °C}
The ultrasonic sensor is used to measure distances by using sound waves. The distance is measured based on the speed of sound wave and on the time it takes to reach the object and bounce back. The following formula is used:
\text{Distance}=\text{speed of sound}\times \frac{\text{Time}}{2}
Time is divided by 2 as the initial time calculated is from the sensor to the object and back. Since the speed of sound is 343 \frac{m}{s} or 34300 \frac{cm}{s}, then the distance is calculated using directly the simplified formula:
\text{Distance[cm]}=17150\times \text{Time[s]}
This formula is used in the code in order to get proper data collected from the sensor. The code also includes a screen reading, where the distance of any object from the sensor is displayed on the screen.