Accelerometer¶
Description¶
The accelerometer is a device used to measure velocity of a moving object between two distant points and its acceleration. It is designed and constructed based on the engineering design process that is followed in the Coder-Maker program.
Learning Objectives¶
The accelerometer is a device that helps students learn and apply the following:
Coding, electronics, and physical science through a hands-on application that can be directly related to real life situations.
Motion concepts of the science of linear motion, mainly velocity and acceleration:
- Initial and final velocity
- Average velocity
- Time
- Acceleration
The implementation of the engineering design process
Application of theoretical concepts to real life situations
Materials Needed¶
- Raspberry Pi with micro SD card with Raspbian
- One ultrasonic sensor
- Jumper wires
- Resistors 300Ω or similar
- Breadboard
- Glue gun and glue sticks
- Cutter blades or scissors
- Thin sturdy cardboard (poster or portfolio cardboard)
Setup and Functionality¶
The accelerometer is a simple device that is programmed on the Raspberry Pi and uses an ultrasonic sensor to collect data of a moving object. When an object is moving towards the sensor, the program starts collecting data about the distance travelled over a defined period of time. Using this data, it will calculate the initial and final velocity of the object in this time interval, and from the velocity and time values, it calculates acceleration. Resistors of 300Ω or similar ones are used in this setup. This will ensure protection of the GPIO on the Raspberry Pi and at the same time all components connected to them will still work properly. As shown in the diagrams below, the accelerometer can be constructed in two different ways. One setup (figure 1) is designed to measure acceleration using one ultrasonic sensor. This setup enables the sensor to measure the acceleration of an object that is moving towards. The program codes for calculation of acceleration and gives a reading every two seconds. The average acceleration over a certain time interval is considered to be the average acceleration value. The other setup (figure 2) is designed to measure the acceleration of an object moving across the sensors. In other words, the moving object will pass in front of sensor one first and then in front of sensor two. In this setup, the start position has to be set at a 15 cm distance from sensor one.
Circuitry and Electronics¶
The digrams below show all the wiring and connections of all the componenets in the setups of both accelerometers:
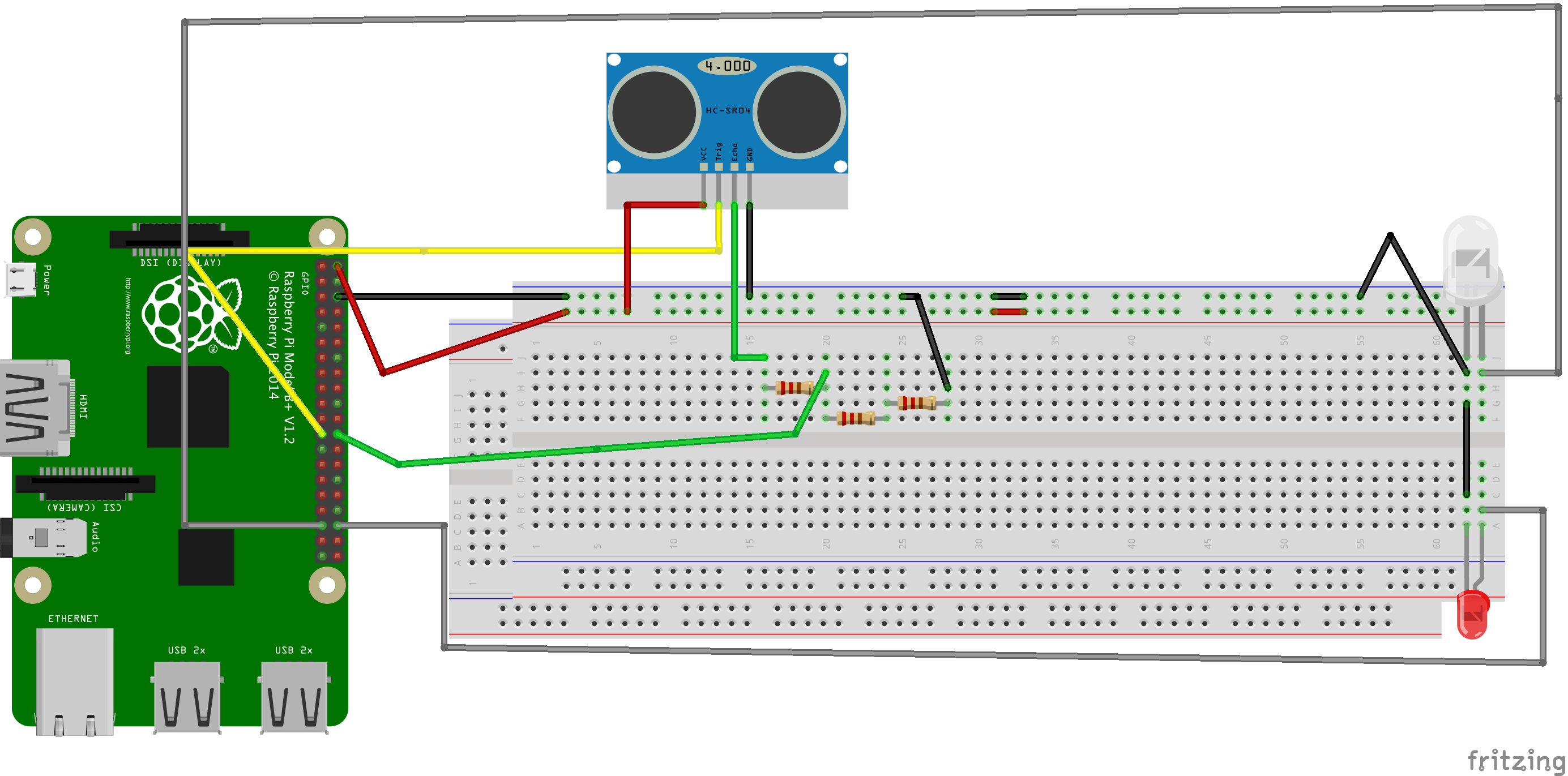
Figure 1: shows the wiring and connections of one ultrasonic sensor to the Raspberry Pi.
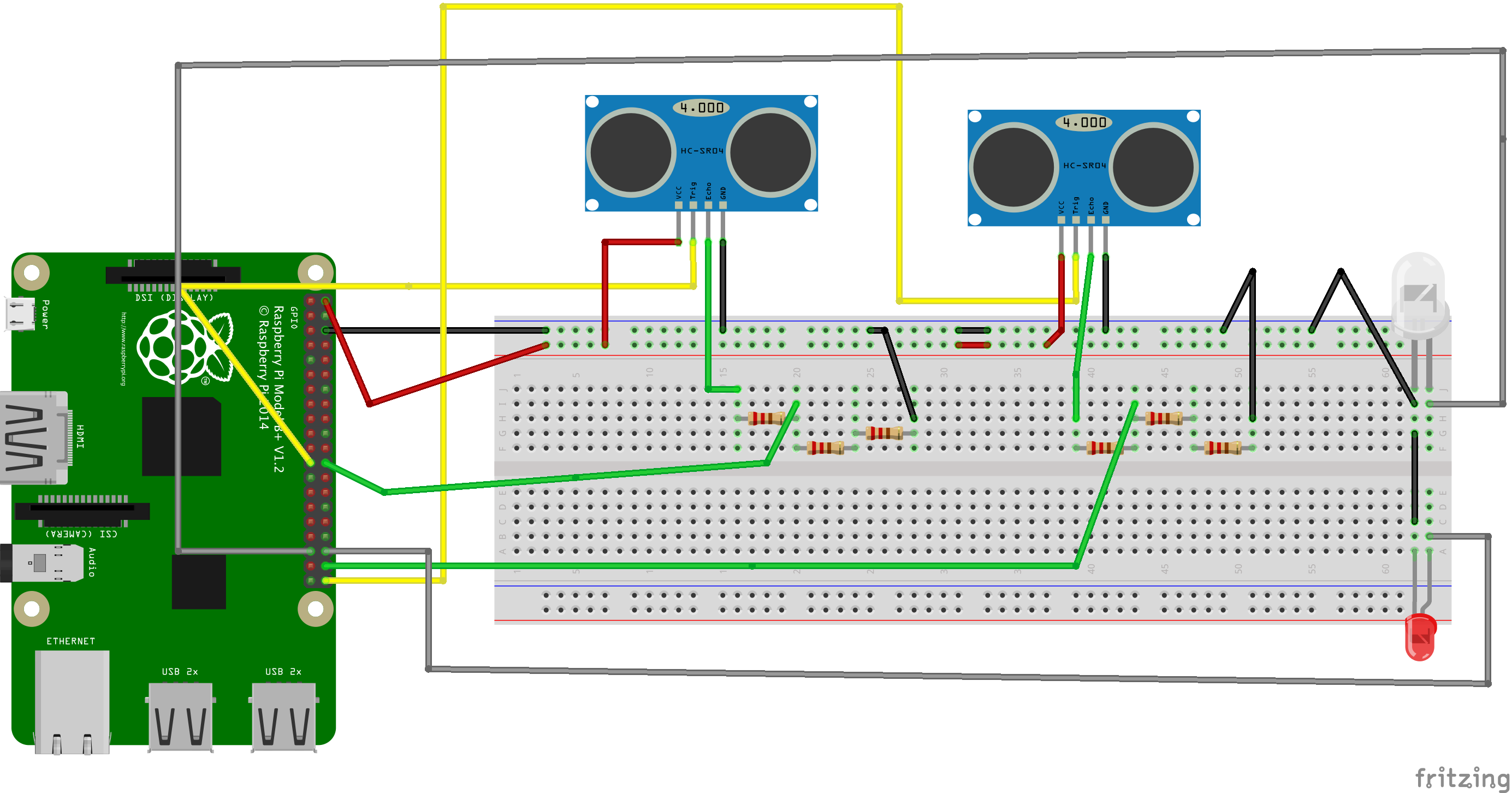
Figure 2: shows the wiring and connections of two ultrasonic sensors to the Raspberry Pi.
Warning
The use of resistors is important for the protection of GPIO on the raspberry Pi. The value of the resistors should be properly chosen based on the components used to ensure proper functioning (see above).
Programming¶
The accelerometer has been programmed using Python language:
Code of the accelerometer that is using one ultrasonic sensor:
import RPi.GPIO as GPIO
import time
import sys
Echo1 = 24
Trig1 = 23
Echo2 = 38
Trig2 = 40
# the distance between starting point-sensor1 and between sensor1-sensor2
Distance = 15
greenLed = 35
redLed = 36
def floatingPointsEquals(a, b, rel_tol=1e-09, abs_tol=0.0):
return abs(a-b) <= max(rel_tol * max(abs(a), abs(b)), abs_tol)
def sensorReading(TRIG, ECHO):
GPIO.output(TRIG, False)
time.sleep(0.5)
GPIO.output(TRIG, True)
time.sleep(0.0001)
GPIO.output(TRIG, False)
start = time.time()
while GPIO.input(ECHO) == 0:
start = time.time()
while GPIO.input(ECHO) == 1:
stop = time.time()
elapsed = stop - start
distance = elapsed * 34000
distance = distance / 2
return distance
def AccelerationWithOneSensor(): # object is perpendicular and v0 = 0
GPIO.output(redLed, True)
time.sleep(3)
GPIO.output(redLed, False)
GPIO.output(greenLed, True)
print ''
start = time.time()
distance = sensorReading(Trig1, Echo1)
while distance > 11:
distance = sensorReading(Trig1, Echo1)
stop = time.time()
velocity = Distance / (stop - start)
print 'elapsed ', stop - start, ' s'
print 'velocity ', velocity, 'cm/s'
GPIO.output(greenLed, False)
acc = velocity/(stop-start)
print 'acceleration ', acc, 'cm/s^2'
return acc
def AccelerationWithOneSensorObjectParallelToSensor():
"""measure distance every 2 seconds."""
GPIO.output(redLed, True)
time.sleep(3)
GPIO.output(redLed, False)
GPIO.output(greenLed, True)
print ''
print 'object need to be at maximum distance of 150 cm of the sensor'
isSet = False
while not isSet:
if sensorReading(Trig1, Echo1) < 150:
isSet = True
print 'Readings will begin'
else:
print 'please put the object at maximum 150cm of the sensor'
distance1 = sensorReading(Trig1, Echo1)
print 'distance1 ', distance1, ' cm'
time.sleep(2)
distance2 = sensorReading(Trig1, Echo1)
print 'distance2', distance2, ' cm'
time.sleep(2)
distance3 = sensorReading(Trig1, Echo1)
print 'distance3 ', distance3, ' cm'
print ''
v1 = (distance1 - distance2) / 2
print 'elapsed Time1', 2, 's'
print 'Distance1 ', distance1-distance2, ' cm'
print 'velocity1 ', v1, ' cm/s'
print ''
v2 = (distance2 - distance3)/2
print 'elapsed time2', 2, ' s'
print 'Distance2 ', distance2-distance3, ' cm'
print 'velocity2 ', v2, ' cm/s'
acc = (v2 - v1) / (4-2)
GPIO.output(greenLed, False)
print 'acceleration ', acc, ' cm/s^2'
def AccelerationWithOneSensorObjectParallelToSensorUntilItStops():
# get the average of acceleration until object stops.
stops = 0
i = 0
acceleration = 0
while stops == 0:
distance1 = sensorReading(Trig1, Echo1)
time.sleep(2)
distance2 = sensorReading(Trig1, Echo1)
if (floatingPointsEquals(distance2, distance1)):
stops = 1
else:
v1 = (distance2 - distance1)/2
time.sleep(2)
distance3 = sensorReading(Trig1, Echo1)
if (floatingPointsEquals(distance3, distance2)):
stops = 1
else:
v2 = (distance3 - distance2)/2
acceleration += (v2 - v1)/2
i = i + 1
acceleration = acceleration/i
print 'acceleration ', acceleration, 'cm/s^2'
try:
GPIO.setmode(GPIO.BOARD)
GPIO.setwarnings(False)
GPIO.setup(Trig1, GPIO.OUT)
GPIO.setup(Echo1, GPIO.IN)
GPIO.setup(Trig2, GPIO.OUT)
GPIO.setup(Echo2, GPIO.IN)
GPIO.setup(greenLed, GPIO.OUT)
GPIO.setup(redLed, GPIO.OUT)
_in2 = raw_input('the object is moving parallel(1) or perpendicular (2)')
try:
numeric2 = int(_in2)
except:
print 'wrong input'
raise SystemExit
if numeric2 < 1 or numeric2 > 2:
print 'wrong input'
raise SystemExit
if numeric2 == 1:
_in3 = raw_input('do you want to calculate acceleration until '
'object stops ? (1) yes or (2) no')
try:
numeric3 = int(_in3)
if numeric3 < 1 or numeric3 > 2:
print 'wrong input'
raise SystemExit
if numeric3 == 1:
AccelerationWithOneSensorObjectParallelToSensorUntilItStops()
if numeric3 == 2:
AccelerationWithOneSensorObjectParallelToSensor()
except:
print 'wrong input'
raise SystemExit
if numeric2 == 2:
AccelerationWithOneSensor()
except KeyboardInterrupt:
GPIO.output(redLed, False)
GPIO.output(greenLed, False)
sys.exit(0)
GPIO.cleanup()
Code of the accelerometer that is using two ultrasonic sensors:
import RPi.GPIO as GPIO
import time
import sys
Echo1 = 24
Trig1 = 23
Echo2 = 38
Trig2 = 40
# the distance between starting point-sensor1 and between sensor1-sensor2
Distance = 15
greenLed = 35
redLed = 36
def sensorReading(TRIG, ECHO):
GPIO.output(TRIG, False)
time.sleep(0.5)
GPIO.output(TRIG, True)
time.sleep(0.0001)
GPIO.output(TRIG, False)
start = time.time()
while GPIO.input(ECHO) == 0:
start = time.time()
while GPIO.input(ECHO) == 1:
stop = time.time()
elapsed = stop - start
distance = elapsed * 34000
distance = distance / 2
return distance
def AccelerationWithTwoSensors():
GPIO.output(redLed, True)
time.sleep(3)
GPIO.output(redLed, False)
GPIO.output(greenLed, True)
print ''
start = time.time()
distance = sensorReading(Trig1, Echo1)
while distance > 11:
distance = sensorReading(Trig1, Echo1)
stop = time.time()
velocity = Distance / (stop - start)
print ''
print('distance between sensors and between sensor1 '
'and start point {} cm'.format(Distance))
print ''
print 'elapsed time', stop - start, ' s'
print 'v1', velocity, ' cm/s'
print ''
distance2 = sensorReading(Trig2, Echo2)
stop2 = stop + 0.001
while distance2 > 11:
distance2 = sensorReading(Trig2, Echo2)
stop2 = time.time()
GPIO.output(greenLed, False)
velocity2 = 2 * Distance / (stop2 - start)
print 'elapsed2', stop2-start, ' s'
print 'v2', velocity2, 'cm/s'
print ''
acc = (velocity2 - velocity) / (stop2 - stop)
print 'acceleration', acc, 'cm/s^2'
return acc
try:
GPIO.setmode(GPIO.BOARD)
GPIO.setwarnings(False)
GPIO.setup(Trig1, GPIO.OUT)
GPIO.setup(Echo1, GPIO.IN)
GPIO.setup(Trig2, GPIO.OUT)
GPIO.setup(Echo2, GPIO.IN)
GPIO.setup(greenLed, GPIO.OUT)
GPIO.setup(redLed, GPIO.OUT)
time.sleep(1)
AccelerationWithTwoSensors()
except KeyboardInterrupt:
GPIO.output(redLed, False)
GPIO.output(greenLed, False)
sys.exit(0)
GPIO.cleanup()
Science Concepts and Skills:¶
The accelerometer helps students learn and apply the Engineering Design Process, learn how to connect electric and electronic circuits, work in teams, solve problems, and relate their learned concepts to real life situations.
The accelerometer can be simply constructed by students for their use in the physics classroom. It helps them better understand the concepts of velocity, time, distance, and acceleration. It also helps them use proper units of measurement.
The following formulas can be applied to measure velocity and acceleration based on the reading values of time and distance as recorded by the ultrasonic sensor. This could be done as part of the code where the program gives direct reading of acceleration on the screen, or manually by coding for readings of time and distance and calculations are done manually:
Calculating Velocity:¶
Velocity is the change in distance in relation to time. To calculate velocity, the following formulas can be used:
- The initial velocity of an moving object: V_0 = \frac{d_1-d_0}{t_1-t_0}
- The final velocity between the start position and the final position reached: V_1=\frac{d_2-d_1}{t_2-t_1}
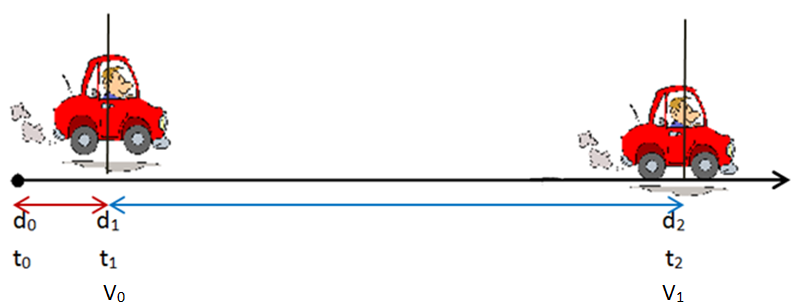
Figure 3: Shows the motion of a car along a horizontal plane. It represents figure 1 situation.
In figure 1, if the car started moving from the dot on the left of the line, then its acceleration needs to be calculated between the two positions (d_1 and d_2), then V_0 is not equal to zero and it needs to be calculated using the formula above. If the object is starting from rest, then d_0=0, t_0=0, and V_0 =0.
Calculating acceleration:¶
Acceleration is the change in velocity of a moving object with respect to time. It can be calculated using the formula below:
a = \frac{V_1-V_0}{t_2-t_1}
The same concept applies to figure 2 setup; however, the start distance is set to 15 cm from sensor one.