L293D Motor Driver Integrated Circuit (Student Version)¶
Description¶
L293D is an integrated circuit (IC) that is used as a motor driver. It allows the user to drive the motors in two directions, clockwise and counter-clockwise. Controlling the rotation of the motor in two directions is done by reversing the current. L293D allows the user to do that. It can operate two motors at one time independently. L293D also allows us to connect an external power supply to operate the motors.
Materials Needed:¶
- Raspberry Pi with micro SD card and Raspbian
- One or two 6V simple DC motors
- 4*1.5V batteries
- IC 293D
- Jumper wires
- Breadboard
Diagram¶
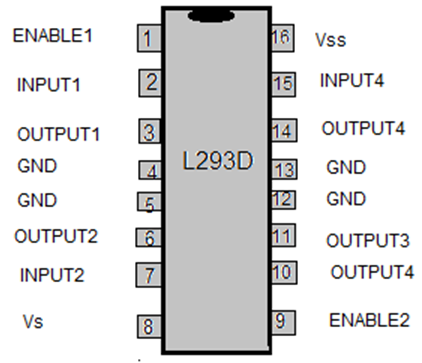
Figure 1: shows a labeled diagram of the pins on IC L293D
Please note that the Vs is connected to the positive pole of the external power supply, the Vss to the 5V Vcc pin of the Raspberry Pi. The ground pins on the IC need to be commonly connected to the ground of the Raspberry Pi and the external power supply. Please refer to the schematic diagrams, figures 2 and 3 below for more details.
Circuitry:¶
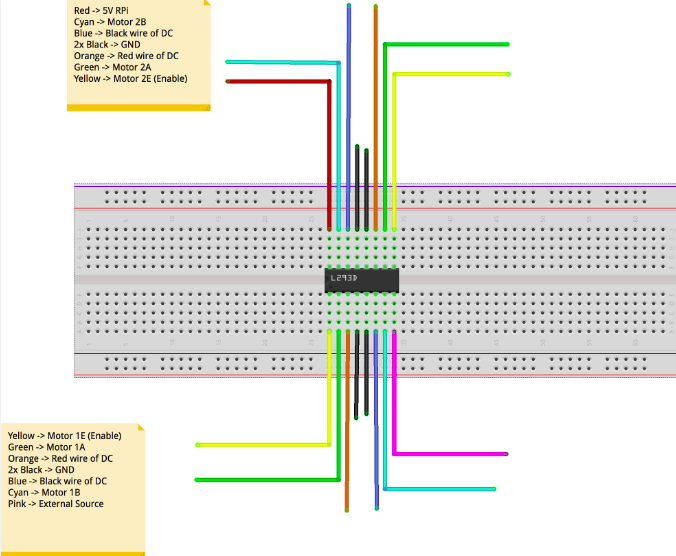
Figure 2: The diagram above shows the wiring of the L293D
Warning
Proper attention should be given to the wiring of the IC L293D. Make sure to follow the diagram above. In addition, changing the polarity of the battery will cause the current to reverse. This will lead to the overheating and damage of the IC. If this happens, the battery needs to be disconnected. Avoid touching the IC as it might cause skin burn.
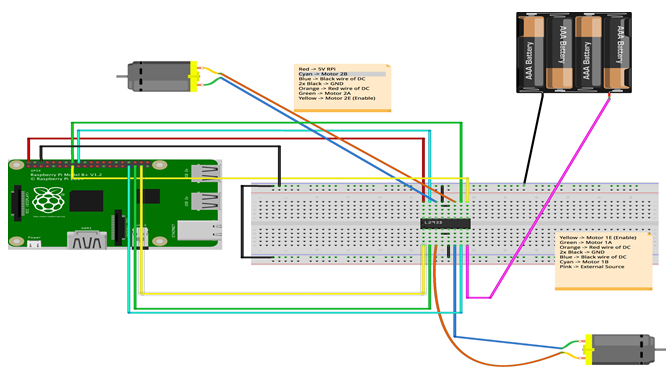
Figure 3: The diagram above shows the connections of L293D to the Raspberry Pi, the external power supply, and one or two motors.
Programming¶
The script below can be used to control one motor:
Controlling One Motor:
import RPi.GPIO as GPIO
import time
motor1A = 35
motor1B = 33
motor1E = 37
GPIO.setmode(GPIO.BOARD)
GPIO.setup(motor1A, GPIO.OUT)
GPIO.setup(motor1B, GPIO.OUT)
GPIO.setup(motor1E, GPIO.OUT)
def clockwise():
GPIO.output(motor1A, False)
GPIO.output(motor1B, True)
GPIO.output(motor1E, True)
def counterClockwise():
GPIO.output(motor1A, True)
GPIO.output(motor1B, False)
GPIO.output(motor1E, True)
# As we can see to turn on the motor Motor1E is alway True/GPIO.HIGH
# The Direction of the Motor will be controlled by MotorA/B
def stop():
"""In order to turn off the motor, we have to disable it in order to do
that we need to stop sending current to the L293D on Motor1E port.
"""
GPIO.output(motor1E, False)
try:
print "Turning the motor Clockwise"
clockwise()
time.sleep(2)
print "Turning the motor CounterClockwise"
counterClockwise()
time.sleep(2)
print "Stopping the motor"
stop()
except KeyboardInterrupt:
stop()
Controlling Two Motors:*
import RPi.GPIO as GPIO
import time
motor1A = 35
motor1B = 33
motor1E = 37
motor2A = 16
motor2B = 18
motor2E = 15
GPIO.setmode(GPIO.BOARD)
GPIO.setup(motor1A, GPIO.OUT)
GPIO.setup(motor1B, GPIO.OUT)
GPIO.setup(motor1E, GPIO.OUT)
GPIO.setup(motor2A, GPIO.OUT)
GPIO.setup(motor2B, GPIO.OUT)
GPIO.setup(motor2E, GPIO.OUT)
def backward():
# in order to move BACKWARD Motor1 has to turn Clockwise
GPIO.output(motor1A, False)
GPIO.output(motor1B, True)
GPIO.output(motor1E, True)
# in order to move BACKWARD Motor2 has to turn CounterClockwise.
GPIO.output(motor2A, True)
GPIO.output(motor2B, False)
GPIO.output(motor2E, True)
def forward():
# in order to move FORWARD Motor1 has to turn CounterClockwise.
GPIO.output(motor1A, True)
GPIO.output(motor1B, False)
GPIO.output(motor1E, True)
# in order to move FORWARD Motor2 has to turn Clockwise.
GPIO.output(motor2A, False)
GPIO.output(motor2B, True)
GPIO.output(motor2E, True)
def turnClockwise():
GPIO.output(motor1A, True)
GPIO.output(motor1B, False)
GPIO.output(motor1E, True)
GPIO.output(motor2A, False)
GPIO.output(motor2B, True)
GPIO.output(motor2E, True)
def turnCounterClockwise():
GPIO.output(motor1A, False)
GPIO.output(motor1B, True)
GPIO.output(motor1E, True)
GPIO.output(motor2A, False)
GPIO.output(motor2B, True)
GPIO.output(motor2E, True)
def stop():
"""In order to turn off the motor, we have to disable it, to do that
we need to stop sending current to the L293D on Motor(1/2)E port.
"""
GPIO.output(motor1E, False)
GPIO.output(motor2E, False)
try:
print "go Forward"
forward()
time.sleep(5)
print "go Backward"
backward()
time.sleep(5)
print "turn clockwise"
turnClockwise()
time.sleep(5)
print "turn counter-clockwise"
turnCounterClockwise()
time.sleep(5)
print "Stop"
stop()
except KeyboardInterrupt:
stop()