Variables¶
What is a variable?¶
A variable describes a place to store information. This information can be a number, a character, a list of characters, etc.
A way of picturing variables, is to imagine a box. This box has a label, for instance ping in the example below. The content of the box is the information we want to store, in the example the number 5.
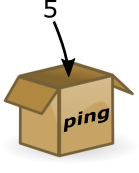
How to use variables in Python?¶
How can we store the number 5 in a box called ping in Python?
In The Python Shell it is a simple as this:
>>> ping = 5
But… what can we do with this variable, what for do we want to store a value behind a label?
We could try to read the value of the variable by using its label, and then print it, like so:
>>> print(ping)
The whole idea of a variable is that its value varies, which means that we put something different in the box. The label that is written on the box however stays the same.
Have a look at this example and try it yourself! Which value appears when you type print(ping)?
Step 1 | |
>>> ping = 5
|
![]() |
Step 2 | |
>>> ping = 3
|
![]() |
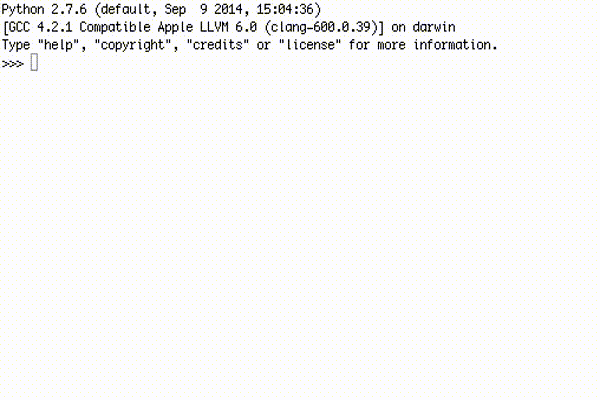
Use variables to do calculations¶
Now that you now how to use variables, there is no limit to what you can do with them. For instance, calculations!
Let us first create two variables with different values: ping and pong.
>>> ping = 5
|
![]() |
>>> pong = 7
|
![]() |
Have a look at the example below that shows what we can do with theses variables, and try it out yourself! For instance:
- Add, multiply or substract the variables ping and pong
- Store the result in a new variable called pingpong
- Compare ping and pong with smaller than (<), bigger than (>)* or equals (==)
- …
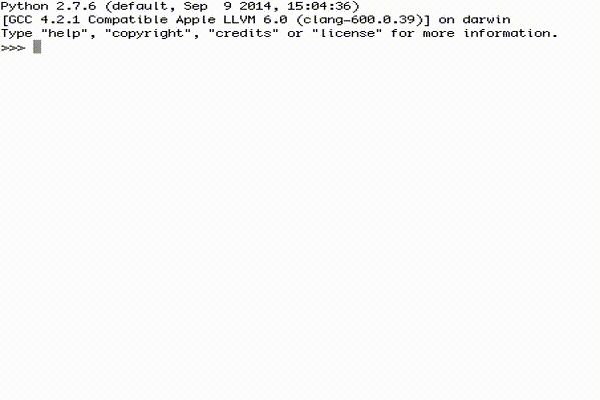
Use variable to write programs¶
Variables are a central concept when writing computer programs. An example that introduces variables in a program is given here: Introducing Variables.