3. Python Primer - Part 2¶
3.1. Talking to your Program¶
As usual, we will start with a small example. Run the following code bellow and type in different values.
1 2 3 4 5 6 7 8 9 10 11 | from turtle import fd, lt
angle = 60
print("Hi, I am Turtle, at your command!")
while True:
steps = raw_input("Tell me how many steps to go: ")
lt(angle)
fd(int(steps))
|
From the above code, can you deduce which functions allow you to interract with your program?
- print()
- Allows you to display a message on the Terminal. The message can be composed by text or a value, or a combination.
- input()
- Not only displays a message to the Terminal, but also reads what the user types in! In our simple example, the number of steps is read and stored in the variable steps.
3.2. Challenge 1¶
Play with the input and output function and develop a small program, which requires interaction with the user!
Here is a small example:
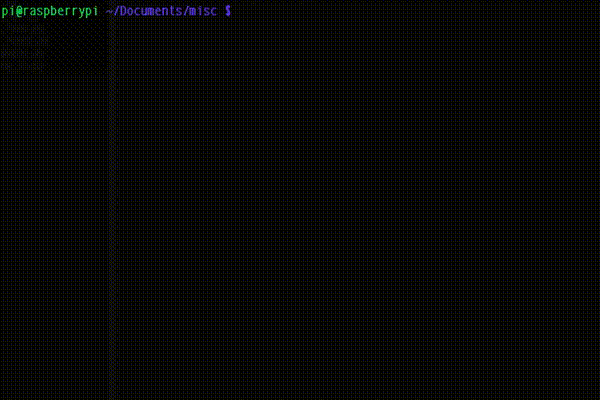
As inspiration, here is the code as well:
1 2 3 4 5 6 7 | import datetime
while(1):
now = datetime.datetime.now()
age = input("How old are you? ")
birth_year = now.year - age
print "You were born in", birth_year
|
Tip
No programmer knows all functions and how to use them by heart.
Therefore, keep in mind that Google is your friend!
Note
In both examples above, an endless loop was used: while(1).
‘1’ in programming means true, as opposed to ‘0’ which means false.
Setting the loop condition to 1, means that it is always true and will go on… forever!
Not very clear yet?
Let us learn more about conditions in the next section, and everything will make more sense.
3.3. Making Decisions¶
3.3.1. Yes or No?¶
Part of our human intelligence is based on our capacity to take decisions, given certain conditions that surround us, and act accordingly.
Does the example bellow look familiar?

The idea is to give part of this intelligence to the computer program, so that it can make decisions on its own!
Note
In programming languages, this is done with conditional statements: IF - THEN - ELSE
Block | Description |
---|---|
IF | Checks if the condition is true. |
THEN | If the condition is true, this action is executed. |
ELSE | Otherwise, if the condition is not true, this other this other action is executed. |

Let us illustrate this with our Turtle example from above, we will make it more intelligent.
Do you remember how the turtle would always go left? Annoying, huh?
Why not ask the user which direction he wants to go, left or right?
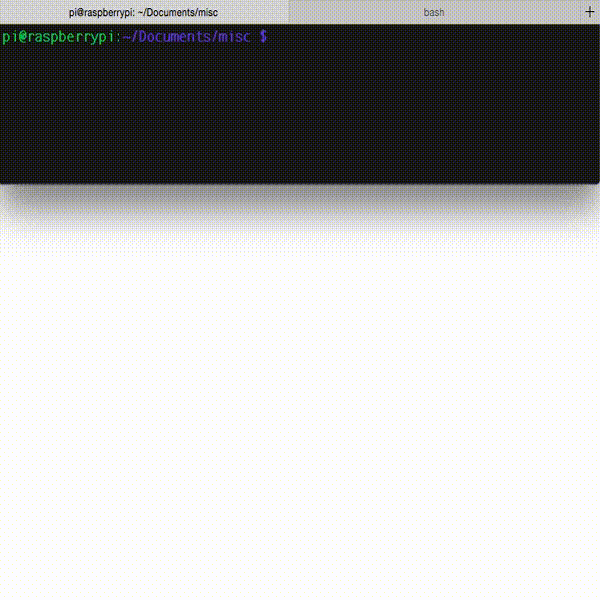
Note
Our turtle is now intelligent enough to go left OR right, depending on the user’s input!
Have a look at how this is done in Python…
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | from turtle import fd, lt, rt
angle = 60
print("Hi, I am Turtle, at your command!")
while True:
steps = raw_input("Tell me how many steps to go: ")
direction = raw_input("And in which direction, left (l) or right (r)? ")
if direction == 'r':
rt(angle)
else:
lt(angle)
fd(int(steps))
|
- In the above example…
- What is condition?
- What happens if the condition is fulfilled?
- What happens if the condition is not fulfilled?
3.3.2. Else, if…¶
Alright, you type in “r”, turlte goes right. You type in “l”, turlte goes left. That is what we wanted.
Have you tried typing in any other letter?
What happens then?
Looking back at the code, can you figure out why?