1. Think like a Programmer¶
1.1. Lea and Max¶
Let us start with an example. Have a look at the animation bellow:
What do you see?
What is happening?
Lea is calling out instructions and Max is following them. We can say that Max is executing Lea’s instructions.
Let us write down the complete sequence of instructions that Lea calls out to Max:
Forward - Turn left - Forward - Turn right - Forward - Turn left - Forward -
Turn left - Forward - Forward - Turn left - Forward 2 steps - Turn left.
We will call the above sequence of instructions code. Notice that Lea used 4 different instructions in her code, let us right them down here:
Forward | Take 1 step forward |
Forward 2 steps | Take 2 steps forward |
Turn left | Turn left by 90 degrees |
Turn right | Turn right by 90 degrees |
1.2. Challenge 1¶
Like Lea and Max, try to crack the code given bellow. Follow the instructions and, on a sheet of paper, move the turtle (black arrow) according to the code. What shape do you obtain?
Forward - Turn right - Forward - Turn left - Forward - Turn left - Forward -
Turn right - Forward - Turn right - Forward - Forward - Turn right - Forward -
Turn right - Forward - Turn left - Forward - Turn left - Forward - Turn right -
Forward - Turn right - Forward - Forward - Turn right.
Note
After executing the code, the turtle is a the same position and looks in the same direction as before you executed the code. From now on, we will talk about start position and start direction.
1.3. Challenge 2¶
Can you write down the code to draw the shape given bellow?
Important
Remember that your turtle should be in the start position and look in the start direction after the execution of your code!
Note
There is more than one correct code to draw this shape, depending for instance in which direction you decide to move your turtle or which instructions you use. Try to find the shortest possible code!
1.4. The Python Turtle¶
Now that you are a master in cracking and writing codes to move any turtle around, it is time to meet Python’s turtle! To do so, open The Python Shell and import the turtle library by typing the following line:
from turtle import *
We are now ready to play with our Python turtle. Type in:
forward(100)
The following window will open:
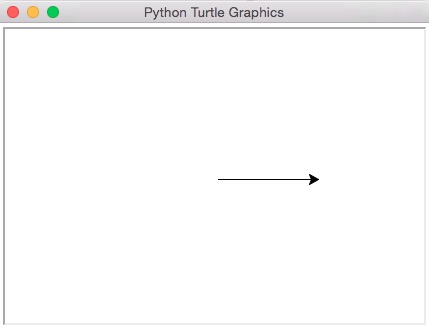
Congratulations, your turtle just executed its first instruction!
Let us have a closer look at the instructions you just typed in:
forward(distance) | Moves the turtle forward by distance |
Note
forward() is called a function and distance is the function parameter.
Can you guess what the other instructions Lea used earlier to guide Max will look like?
Here is a table with Lea’s instructions translated to Python:
Lea and Max | Python Turtle | Description |
---|---|---|
Forward | forward(100) | 1 step forward |
Forward 2 steps | forward(200) | Take 2 steps forward |
Turn left | left(90) | Turn left by 90 degrees |
Turn right | right(90) | Turn right by 90 degrees |
Note
If you want to make bigger or smaller steps, you can replace the distance parameter of the forward(distance) function by any value!
Now that you know all the instructions, can you re-draw Max and Lea’s shape with your turtle?
Here is some help… Make sure to understand this example to be able to solve Challenge 3!
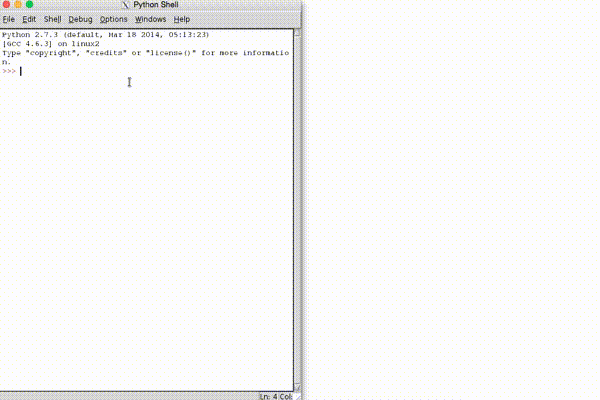
Hint
The following function will clear the screen and place the turtle at its start position: reset()
1.5. Challenge 3¶
As in the example above, draw the shape from Challenge 1 with the Python turtle!
Can you do it for the shape of Challenge 2 as well?
1.6. Write a Python Script¶
You may have noticed that each time you made a mistake, you would have to enter all instructions from scratch. In order to avoid this, we will now create a Python script with our instructions. A script, or program, is simply a sequence of instructions that is saved somewhere on your Pi. When you run a script, the instructions are executed one by one in the order they are written.
Can you write and run the script for Max and Lea’s example?
Here is a possible solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 from turtle import forward, left, right, exitonclick # this line is a comment and will be skipped when executing the script forward(100) left(90) forward(100) right(90) forward(100) left(90) forward(100) left(90) forward(100) forward(100) left(90) forward(200) left(90) # this will close the window when clicking on it exitonclick()
1.7. Challenge 4¶
Try to imagine any basic shape (square, circle, triangle, hexagons, etc…) and then draw it using the turtle!
1.8. More Turtle Functions¶
Now that you are familiar with the functions introduced so far, forward(distance), left(angle), right(angle), try out the functions listed in the table bellow. Can you describe shortly what they do?
Python Turtle | Description |
---|---|
backward(distance) | Makes the turtle go back a distance |
setx(xpos) | Moves the turtle along the same y to a specified x |
sety(ypos) | Moves the turtle along the same x to a specified y |
goto(x,y) | Moves the turtle to a specific x and y |
Tip
You will find all the answers and more turtle functions here:
http://www.eg.bucknell.edu/~hyde/Python3/TurtleDirections.html