2. Python Primer - Part 1¶
2.1. Challenge 1¶
As a repetition from the last lesson, write a Python script that draws an equilateral triangle (all three sides are equal, which results in all 3 angles being equal as well). The triangle should have a side length of 100. Run the script.
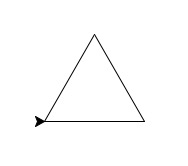
Next, change your script to draw a triangle with the side has length 200. Re-run the script.
Do it again, this time with side=50.
What do you have to change to adjust the size of the triangle?
How many changes do you need to do?
Do you have an idea how you could make the changes easier, faster?
2.2. Introducing Variables¶
Have a look a the two codes shown bellow. Both of them draw an equilateral triangle with side=135.
from turtle import fd, lt, exitonclick
# fd is short for "forward"
# lt is short for "left turn"
# Equilateral triangle with side=135
fd(135)
lt(120)
fd(135)
lt(120)
fd(135)
lt(120)
exitonclick()
|
from turtle import fd, lt, exitonclick
side = 300
fd(side)
lt(120)
fd(side)
lt(120)
fd(side)
lt(120)
exitonclick()
|
How many changes do you need to make in the code on the right side to change the size of the triangle?
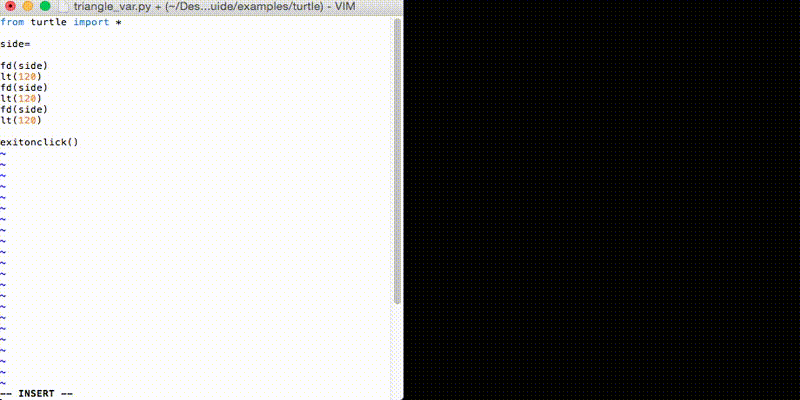
Note
You just learned how to use variables. Once you have assigned a value to a variable (second instruction in the right code), you can use it anywhere in the code. Choosing meaningful names for your variables will help you to write structured and clean code.
Tip
You can find out more about variables here : Variables
2.3. Challenge 2¶
Are you able to write a script drawing the following three triangles? (Hint: side=50,100,200)
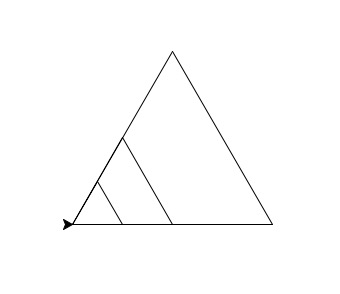
How is your code structured?
Are there parts of the code being repeated?
What changes between two repeated parts?
2.4. The Triangle Function¶
Bellow are two different codes drawing the three triangles given above in the exact same way. Take a few minutes to inspect the two examples.
from turtle import fd, lt, exitonclick
angle = 120
# 1st triangle, side=50
side = 50
fd(side)
lt(angle)
fd(side)
lt(angle)
fd(side)
lt(angle)
# 2nd triangle, side=100
side = 100
fd(side)
lt(angle)
fd(side)
lt(angle)
fd(side)
lt(angle)
# 3rd triangle, side=200
side = 200
fd(side)
lt(angle)
fd(side)
lt(angle)
fd(side)
lt(angle)
exitonclick()
|
from turtle import fd, lt, exitonclick
angle = 120
def triangle(side):
fd(side)
lt(angle)
fd(side)
lt(angle)
fd(side)
lt(angle)
triangle(50)
triangle(100)
triangle(200)
exitonclick()
|
What is the first and obivous difference you see between both codes?
How are the repetions in the left code handled in the right code?
Does it look familiar to something we used before?
Note
We just defined our own Triangle function thanks to the keyword def. Our function takes one parameter, side.
Functions will help you organize your code, especially when you want to re-use parts of the same code.
You can also think of a function as a black box, which will procude a different result depending on the input parameter. You do not necessarily need to know what is inside the black box, unless you write it yourself.
Examples of functions we already know: forward(distance), left(angle), reset(), etc.
Important
Note the indentation after def Triangle(side):. In Python, it is crucial to respect this indentation for your code to run properly!
2.5. Challenge 3¶
Drawing this should be a piece of cake for you by now ;)
Use functions and variables in your code! The side length of the triangles varies from 10 to 100.
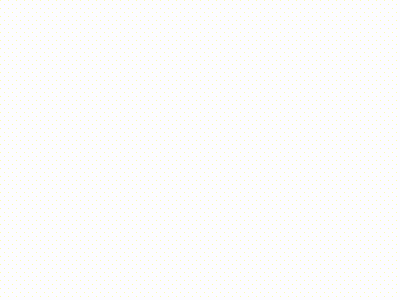
How many times is the function triangle called in your code?
How would you change your code to draw 20 triangles? And a 100? A 1000?
2.6. Repetition is the Key to Success¶
Let us have a look at how we can easily and efficiently handle repetitions in our code using loops. In the example code on the left, a for loop is used.
from turtle import fd, lt, exitonclick
angle = 120
def triangle(side):
fd(side)
lt(angle)
fd(side)
lt(angle)
fd(side)
lt(angle)
triangle(10)
triangle(20)
triangle(30)
triangle(40)
triangle(50)
triangle(60)
triangle(70)
triangle(80)
triangle(90)
triangle(100)
exitonclick()
|
from turtle import fd, lt, exitonclick
angle = 120
def triangle(side):
fd(side)
lt(angle)
fd(side)
lt(angle)
fd(side)
lt(angle)
for i in range(1, 11):
triangle(i*10)
exitonclick()
|
Let us decorticate these two lines:
for i in range(1,11):
Triangle(i*10)
The variable i takes the values in range(1,11), which means from 1 to 10. For each of these values, the Triangle function is called, with the according multiple of 10 as parameter.
Important
Remember the indentation after for i in range(1,11):! Also here, your code will not be executed properly if not indented corretly.
Still not convinced?
Use the Python Tutor and visualize the execution of the following code:
for i in range(0,10):
print i
What happens when you change range(0,10) to range(0,10,2)?
Note
There are different kind of loops: for-loops, while-loops, do-while-loops. Using Internet, can you find out what differs them?
2.7. Challenge 4¶
You have now all the tools in hand to build some really cool stuff! Take the time to look back at what you just learned and apply it with the help of your imagination!
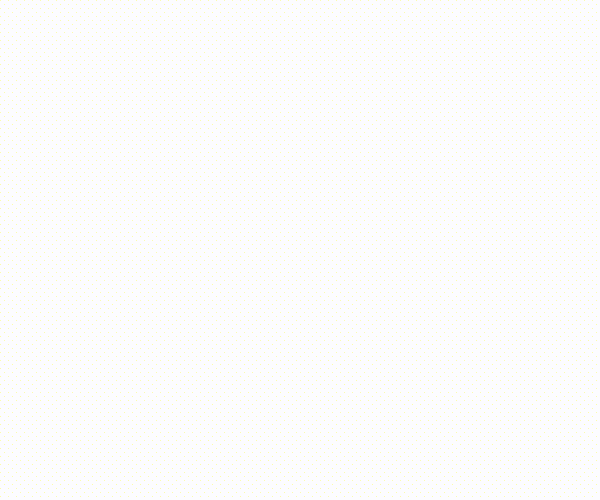
Tip
Some very useful turtle functions are given here:
http://www.eg.bucknell.edu/~hyde/Python3/TurtleDirections.html
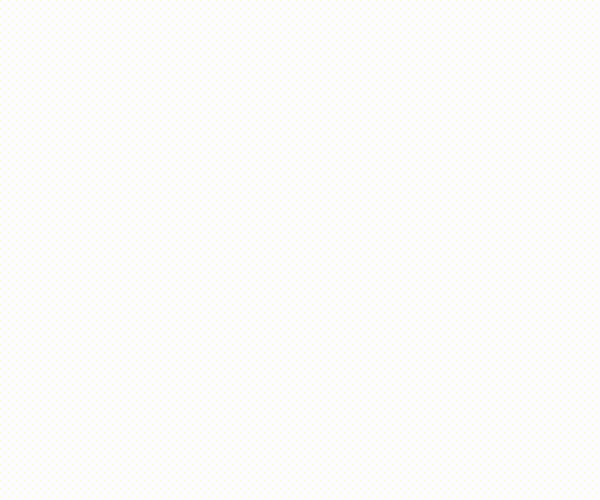
See also
Have a look at the full Turtle documentation: