4. Python Primer - Libraries¶
In this chapter, you will learn about a very powerful and usefull tool: libraries!
You know libraries from school, right? A room filled with a collection of books that you can borrow and use for you school projects. Well, here we are talking about something very similar, but instead of books, we will borrow functions or variables or constants, etc. Basically, all the stuff we already learned. :)
4.1. The “turtle” Library¶
Actually, you have been using libraries all this time, but withoug knowing it! Have a look of the first line of this code example from Python Primer - Part 1:
from turtle import fd, lt, exitonclick
# fd is short for "forward"
# lt is short for "left turn"
# Equilateral triangle with side=135
fd(135)
lt(120)
fd(135)
lt(120)
fd(135)
lt(120)
exitonclick()
Let us have a closer look:
from turtle import fd, lt, exitonclick
Do you remember how we called fd, lt and exitonclick? Yes, exactly, functions! ;)
Important
We import functions from a library in order to use them in our code.
In the example above, the library is “turtle” and the functions “fd”, “lt” and “exitonclick”.
Try the following exercises in the The Python Shell!
4.1.1. Example 1¶
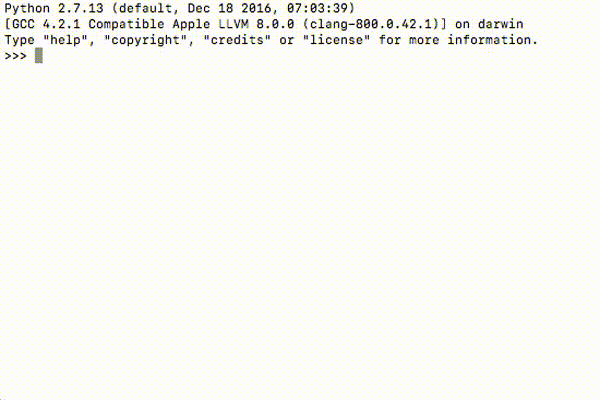
Note
It is good practice to import only the functions we really need in our code, like we did above. However, while developping, it could usefull to import them all, with:
“from turtle import * “
4.1.3. Example 3¶
What happens if you try to import a function that does not exist in the library?
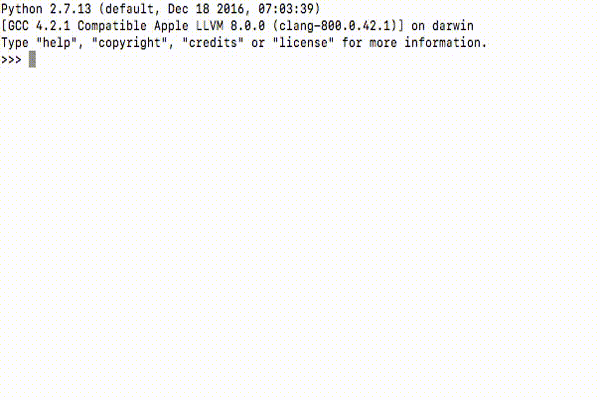
Note
If you do not know whether a function is in the library, you can check the documentation online, for instance for the “turtle” library:
4.2. “Import turtle” versus “from turtle import …”¶
Until now, after important a function from a library, we could use it by simply typing its name.
What happens when you import two functions with the same name, but from different libraries?
Well, to tackle this problem, another way to import a library, is to use:
import turtle
To use a function for this library, you will have to specify from which library you want to use the function:
turtle.fd(100)
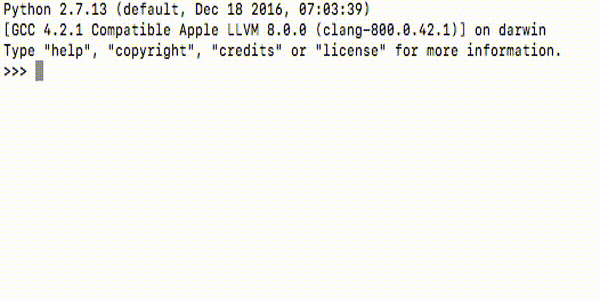
4.3. The “time” Library¶
Imagine that for some reason, you would like to make your turtle draw a square, but it should wait two seconds at each corner before continuing. It would look like:
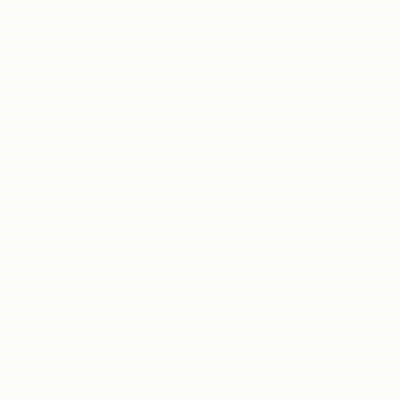
What do you need to make the turtle stop?
Have a look at the code behind the example above. What libraries are used?
Which function tells the turtle to wait two seconds?
import turtle
import time
side = 200
angle = 90
wait = 2
for i in range(0, 3):
turtle.fd(side)
time.sleep(wait)
turtle.lt(angle)
turtle.fd(side)
turtle.lt(angle)
turtle.exitonclick()
Important
The library time contains many very useful time-related functions. A few examples are:
- time.sleep(secs) : suspends the execution of the programm for the given number of seconds
- time.ctime() : gives current local time and date
- time.time() : returns the time in seconds since the epoch as a floating point number
What is the difference between “ctime()” and “time()”?
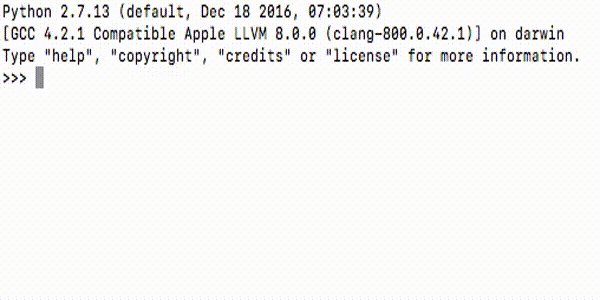
Note
ctime() returns the time and date so that a human can easily read it. time() returns a number of seconds, which does not make a lot of sense for a human, but is much easier to store and use for a computer!
4.4. Measuring time with “time.time()”¶
Let us take the example of the square above.
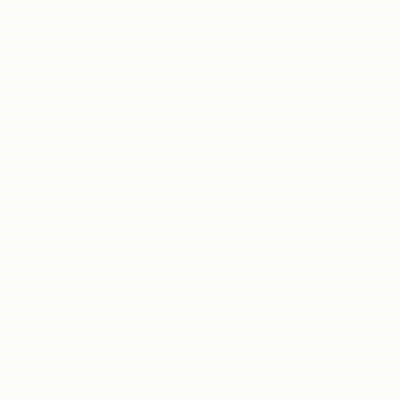
If you were asked to calculate how long the turtle needs, to draw the square, how would you do it?
Have a look at the following code and try to understand how time is measured:
import turtle
import time
side = 200
angle = 90
wait = 2
# store start time:
startTime = time.time()
for i in range(0, 3):
turtle.fd(side)
time.sleep(wait)
turtle.lt(angle)
turtle.fd(side)
turtle.lt(angle)
# store end time:
endTime = time.time()
# calculate the difference:
elapsedTime = endTime-startTime
# print the difference:
print('It took {} seconds to draw the square'.format(elapsedTime))
turtle.exitonclick()
Important
To compute the elapsed time, store the current time with time.time() at two places in the code and calculate the difference.
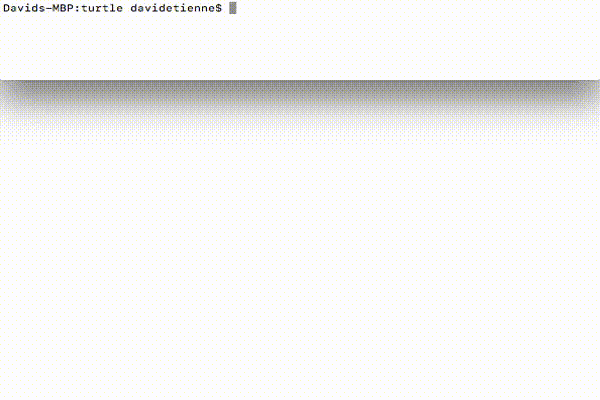