9. Light Intensity Sensor¶
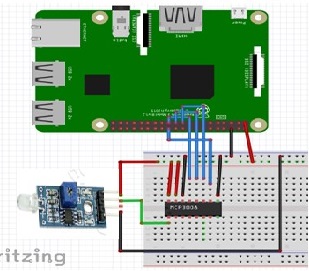
Light Intensity sensor (photoresistor) generates an output voltage, proportional to the light intensity detected.
As light intensity increases, energy increases as well, resulting in bound electrons breaking free and conducting electricity, decreasing resistance, and thus creating a higher voltage.
Warning
Before proceeding with this sensor, you will need to learn about the MCP3008 ADC.
9.1. Connecting the Sensor¶
Light Intensity sensor returns analog signals. Since the Raspberry Pi can only read digital signals, the MCP3008 ADC will be used as ADC(analog-to-digital converter).
Each pin of the MCP3008 needs to be connected to its respective pin on the Raspberry Pi, according to the below image.
9.2. Python Program to Read Light Intensity¶
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 import spidev import time import os # Open SPI bus spi = spidev.SpiDev() spi.open(0,0) # Function to read SPI data from MCP3008 chip # Channel must be an integer 0-7 def ReadChannel(channel): adc = spi.xfer2([1,(8+channel)<<4,0]) data = ((adc[1]&3) << 8) + adc[2] return data # Function to convert data to voltage level: # 3.3 for Voltage used , 1023 for the 10 bits analog pin def ConvertVolts(data,places): volts = (data * 3.3) / float(1023) volts = round(volts,places) return volts # Define sensor channels light_channel = 0 # Define delay between readings delay = 2 while True: # Read the light sensor data light_level1 = ReadChannel(light_channel) light_level=100-(light_level1*100)/1023 light_volts = ConvertVolts(light_level,2) # Print out results print "--------------------------------------------" print("Light: {}% ({}V)".format(light_level,light_volts)) # Wait before repeating loop time.sleep(delay)