7. MCP3008 ADC¶
MCP3008 is the chip that is used with analog sensors in order convert analog signals to digital. Since the Raspberry Pi can only read digital signals, this chip must be used to connect analog sensors to the Raspberry Pi. It has 8 channels on the left side to read the analog input, and output the digital from the right side.
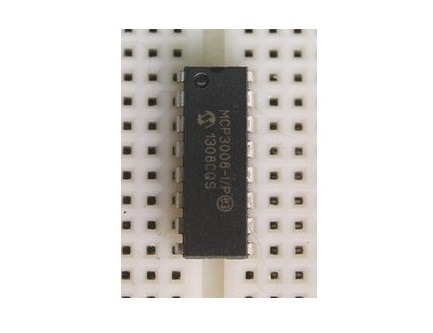
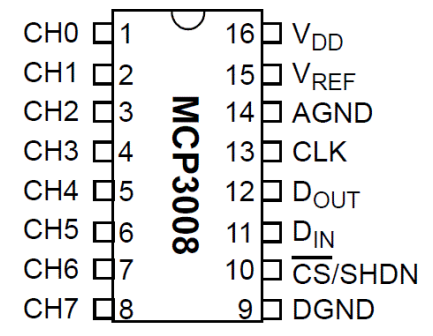
MCP3008 uses SPI (Serial Peripheral Interface bus) which uses spidev library for Python. Therefore, after connecting the MCP, you will need to install the library from Github, and enable SPI.
7.1. Connecting to the Raspberry Pi¶
Press the chip in the middle of the breadboard. Be sure to place it with the half circle indention and dot towards the top. For the sensors to work, 3 pins must be connected.
7.1.1. Option 1 : Hardware SPI¶
Connect the MCP3008 as indicated below, each leg of the chip to its respective pin on the Raspberry Pi. The Hardware SPI is faster but less flexible since it needs specific pins.
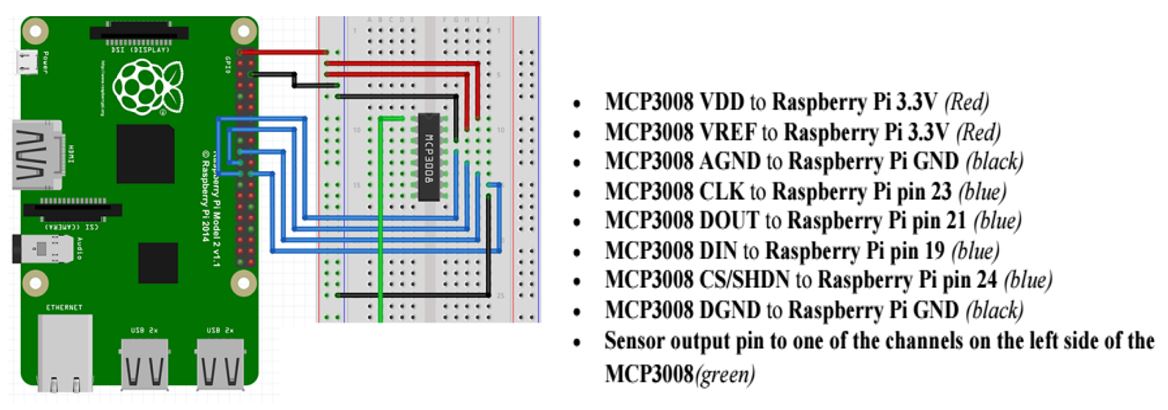
7.1.2. Option 2 : Software SPI¶
Connect the MCP3008 to any four GPIO pins and software SPI to talk to it as shown below. In this example pins 18, 23, 24 and 25 were used.
Enter this code after installing and enabling SPI:
# Software SPI configuration: import Adafruit_MCP3008 CLK = 18 DOUT = 23 DIN = 24 CS = 25 mcp = Adafruit_MCP3008.MCP3008(clk=CLK, cs=CS, miso=MISO, mosi=MOSI)
7.2. Enable SPI & Install Library¶
Before using MCP3008, you must enable SPI in RPi. In terminal:
Type ‘sudo raspi-config’, go to ‘Advanced options’, ‘SPI Enable’, then reboot.
Note
If you are using Raspberry Pi3:
Open applications menu, go to ‘Preferences’, ‘Raspberry Pi Configuration’, ‘ Interfaces’, and check ‘Enable’ next to SPI.
Warning
You must have internet connection on RPi before you perform the upcoming steps.
Type the following codes in the terminal to install the library from Github:
- sudo apt-get update
- sudo apt-get install build-essential python-dev python-smbus git
- cd
- git clone https://github.com/adafruit/Adafruit_Python_MCP3008.git
- cd Adafruit_Python_MCP3008
- sudo python setup.py install
7.3. General Python Code to Control the MCP¶
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | import spidev
import time
delay = 0.5
ldr_channel = 0 #Using channel 0 of the chip
#Create SPI
spi = spidev.SpiDev()
#Opening SPI_PORT 0,and SPI_DEVICE 0
spi.open(0, 0)
def readadc(adcnum):
if adcnum >7 or adcnum<0:
return -1
r=spi.xfer([1, 8 + adcnum << 4 ,0])
data = ((r[1]&3)<<8 + r[2])
return data
#the data returned will be used
#depending on the device/sensor being read
|
We will next see how we can use the MCP with a multitude of sensors to get readings…